Hey everyone, welcome to our new article! Today, we’ll learn on the topic Switch Statement in Dart. Let’s get started!
Switch Statement in Dart
In Dart, a switch
statement allows you to execute one set of instructions from multiple possible sets based on the value of an expression. It is often used as an alternative to multiple if-else
statements, providing a more readable and structured way to handle multiple conditions.
An alternate way to handle control flow, especially for multiple conditions, is with a switch statement. The switch statement takes the following form:

There are a few different keywords, so here are what they mean:
- switch:
- Based on the value of the variable in parentheses, which can be an int, String or compile time constant, switch will redirect the program control to one of the case values that follow.
- case:
- Each case keyword takes a value and compares that value using == to the variables after the switch keyword. You add as many case statements as there are values to check. When there’s a match Dart will run the code that follows the colon.
- break:
- The break keyword tells Dart to exit the switch statement because the code in the case block is finished.
- default:
- If none of the case values match the switch variable, then the code after default will be executed.
The following sections will provide more detailed examples of switch statements.
Replacing else-if chains
Using if statements are convenient when you have one or two conditions, but the syntax can be a little verbose when you have a lot of conditions. Check out the following example:
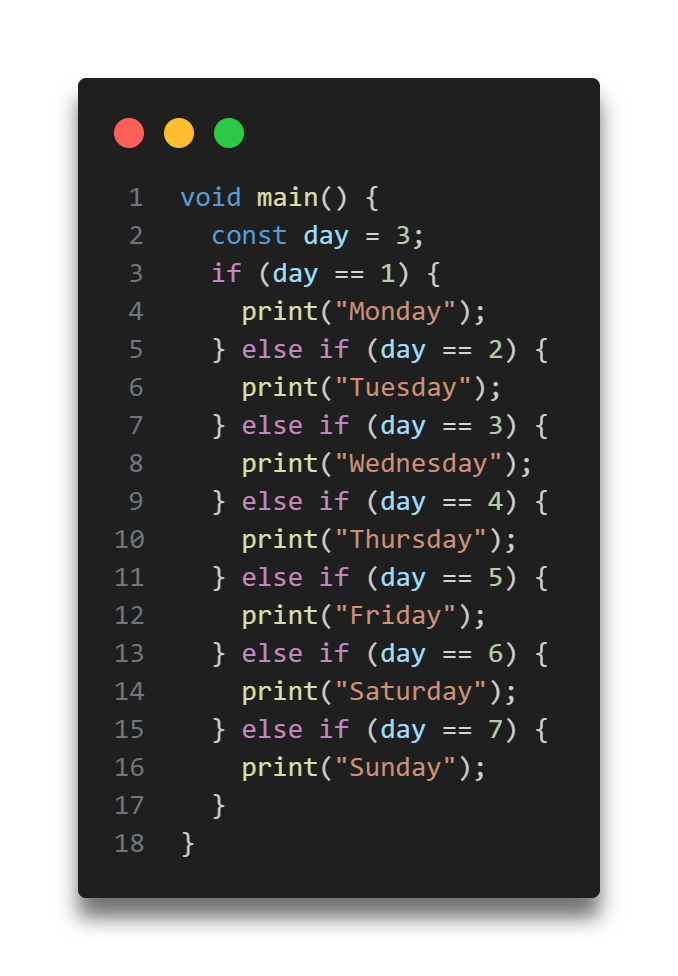
Run that code and you’ll see that it gets the job done – it prints Wednesday as expected. The wordiness of the else-if lines make the code kind of hard to read, though.
In Dart, switch statements don’t support ranges like number > 5. Only == equality checking is allowed. If your conditions involve ranges, then you should use if statements.
Note
Switching on strings
A switch statement also work with strings. Try the following example:

Run the code above and the following will be printed in the console:
Bring an umbrella.
In this example, the “Cloudy” case was completely empty, with no break statement. Therefore, the code “falls through” to the ‘rainy’ case. This means that if the value is equal to either ‘cloudy’ or ‘rainy’, then the switch statement will execute the same case.
Enumerated types
Enumerated types, also known as Enums, play especially well with switch statements, you can use them to define your own type with a finite number of options.
Consider the previous example with the switch statement about weather. You’re expecting weather to contain a string with a recognized weather word. But it’s conceivable that you might get something like this from one of your users:
const weather = 'I like turtles.';
You’d be like, “What? What are you even talking about?”
That’s what the default case was there for – to catch all the weird stuff that gets through. Wouldn’t it be nice to make weird stuff impossible, though? That’s where Enums come in.
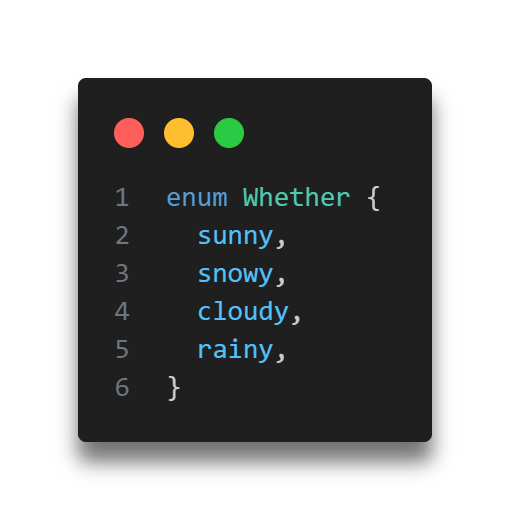
This Enum defines four different kinds of weather. Yes, yes, you can probably think of more kinds than that; feel free to add them yourself. But please don’t make iLikeTurtles and option. Separate each of the values with a comma.
Naming enums
When creating an Enum in Dart, it’s customary to write the Enum name with an initial capital letter, as Weather was written in the example above. The values of an Enum should use lowerCamelCase unless you have a special reason to do otherwise.
Switching on enums
Now that you have the Enum defined, you can use a switch statement to handle all the possibilities, like so:
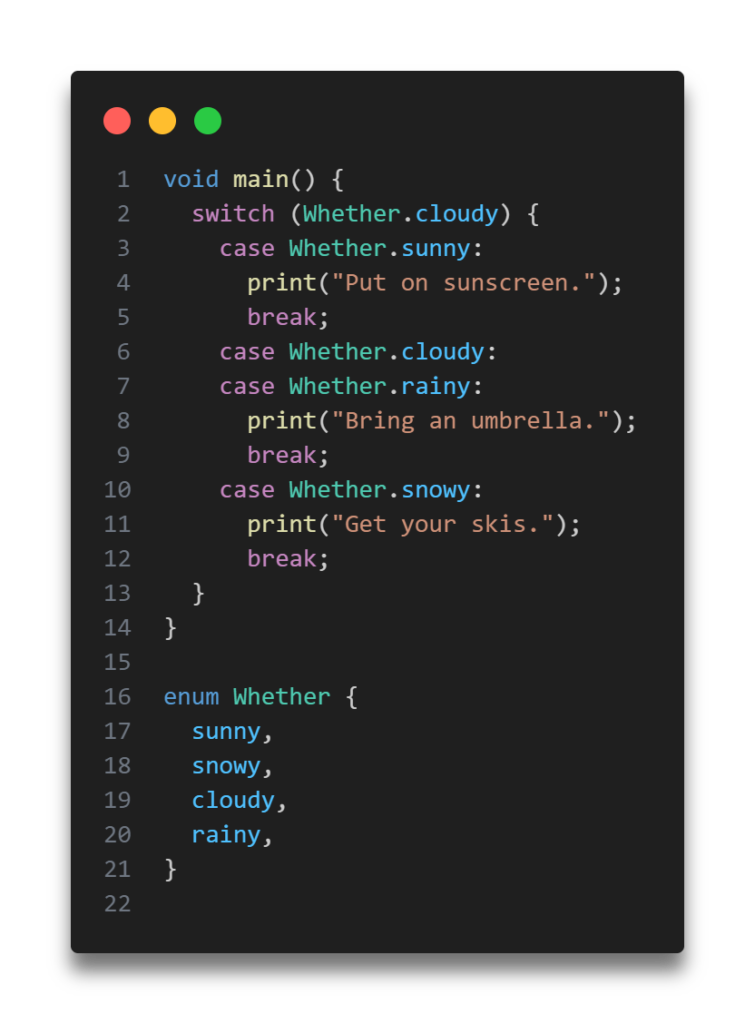
Notice that there was no default case this time since you handle every single possibility. In fact, Dart will warn you if you leave one of the enum items out. That’ll save you some time chasing bugs.
Avoiding the overuse of switch statements
Switch statements, or long else-if chains. can be a convenient way to handle a long list of conditions If you’re a beginning programmer, go ahead and use them; they’re easy to use and understand.
However, if you’re an intermediate programmer and still find yourself using switch statements a lot, there’s good change you could replace some of them with more advanced programming techniques that will make your code easier to maintain. If you’re interested, do a web search for refactoring switch statements with polymorphism and read a few articles about it.
Check out more articles related to Dart Tutorial:
4 thoughts on “Switch Statement in Dart”