Hello Learners! article: Dart Variables and Data Types
If you looking for the documentation or any source that explain you every miner details about dart then you coming on right place.
In this article we will discuss about every details related Dart programming.
At its simplest, computer programming is all about manipulating data, since everything you see on your screen can be reduced to numbers.
Sometimes you represent and work with data as various type of numbers, but other time, the data comes in more complex forms such as text, images and collections.
In your Dart code, you can give each piece of data a name you can use to refer to that piece of data later. The name carries with it an associated type that denotes what sort of data the name refers to, such as text, numbers or a date.
Introduction
Dart is a modern programming language, designed primarily for building fast, scalable, and easily maintainable applications, especially for mobile, web, and desktop platforms.
It’s used widely with Flutter, a popular framework for building cross-platform apps.
Dart was developed by Google with a focus on improving the development of apps. It’s known for:
- Speed: Dart compiles directly into native code mobile apps, making them run quickly.
- Cross-Platform: With Dart, you can write a single codebase that runs on Android, iOS, web, and even desktop.
As its core, Dart is an Object-Oriented Language, meaning everything in Dart is an object. This makes it similar to other languages like Java or C++. It also follows a clear structure:
- Classes: A class is like a blueprint. You define how an object should behave and what attributes it should have.
- Objects: Objects are instance of classes, used to represent real-world things (e.g., a car, user, etc.).
Basic Syntax
The syntax (rules for writing code) of Dart is clean and easy to understand. Here’s an example:
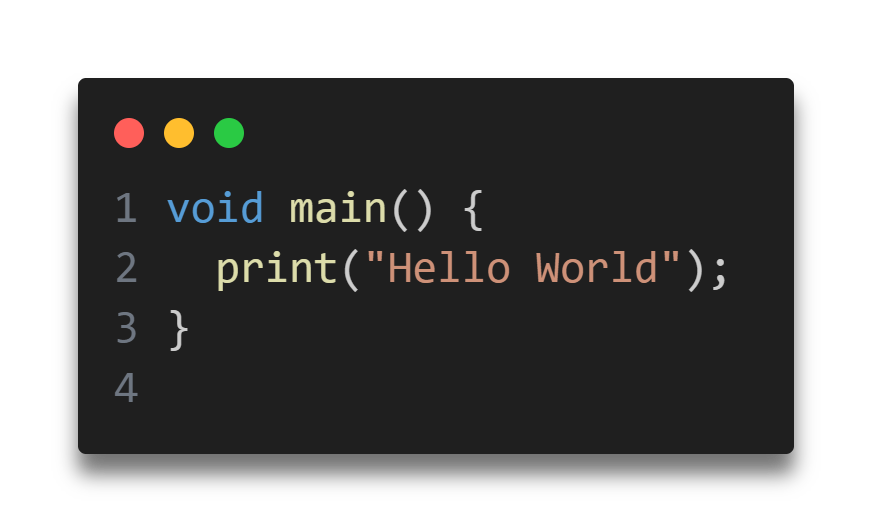
In this simple program:
- void main() is the entry point of every Dart program.
- print() is a function used to display text.
Variables in Dart
Take a look at the following:
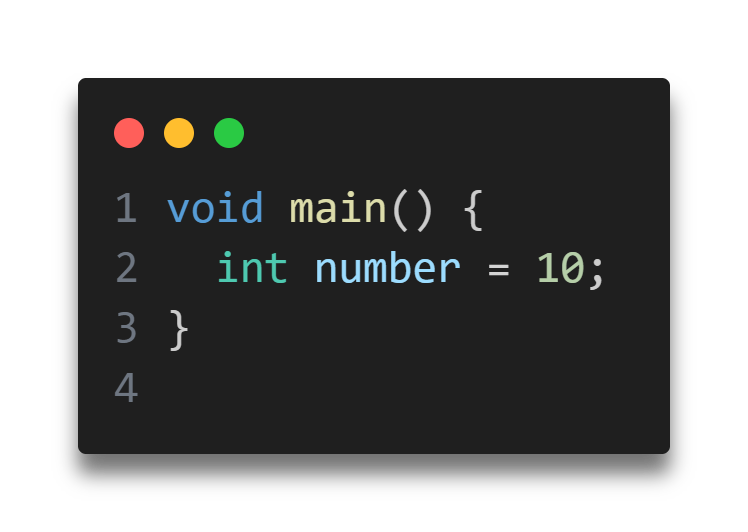
This statements declares a variables called number of type int.
It then sets the value of the variable to the number 10. The int part of the statement is know as a type annotation, which tells Dart explicitly what the type is.
Note: Thinking about = operators.
This equals sign, = , is known as the assignment operator because it assigns a value to a variables. This is different than the equals sign you are familiar with from math.
If you want to change the value of the variable, then you can just give it a different value of the same type:
Dart Variables And Data Types
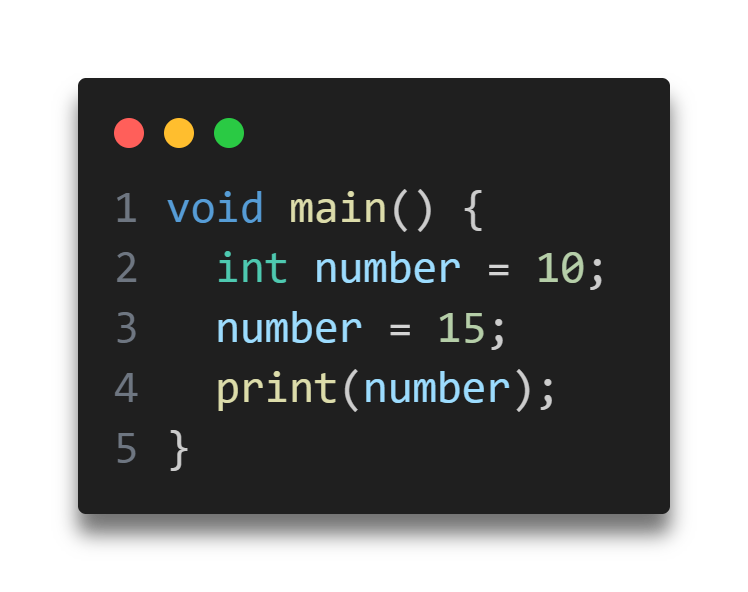
The type int can store integers. The way you store decimal number is like so:
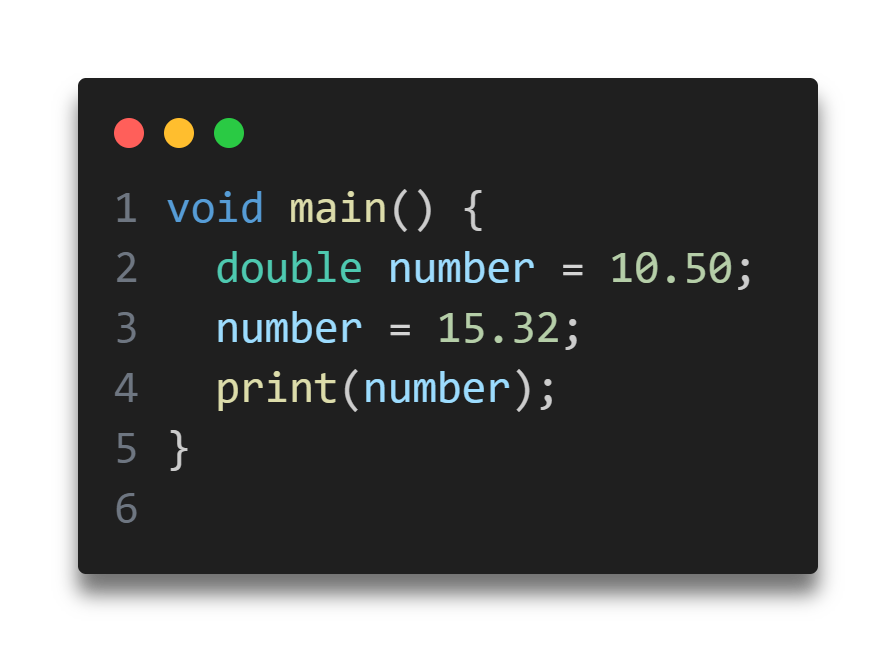
This is similar to the int example. This time, though, the variable is a double, a type that can store decimals with high precision.
For readers who are familiar with object-oriented programming, you’ll be interested to learn that 10, 3.14159 and every other value that you can assign to a variables are objects in Dart. In fact, Dart doesn’t have the primitive variable types that exist in some languages like java.
Everything is an object. Although int and double look like primitives, they’re subclasses of num, which is a subclass of Object. Dart Variables And Data Types
With numbers as objects, this lets you do some interesting things:
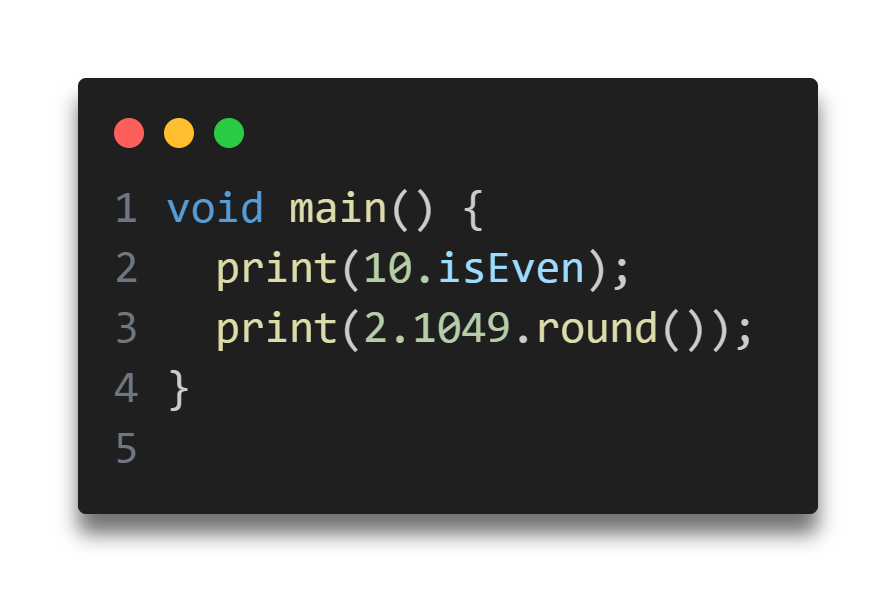
Type safety in Dart Variables And Data Types
Dart is a type-safe language. That means once you tell Dart what a variable’s type is, you can’t change it later. Here’s an example:
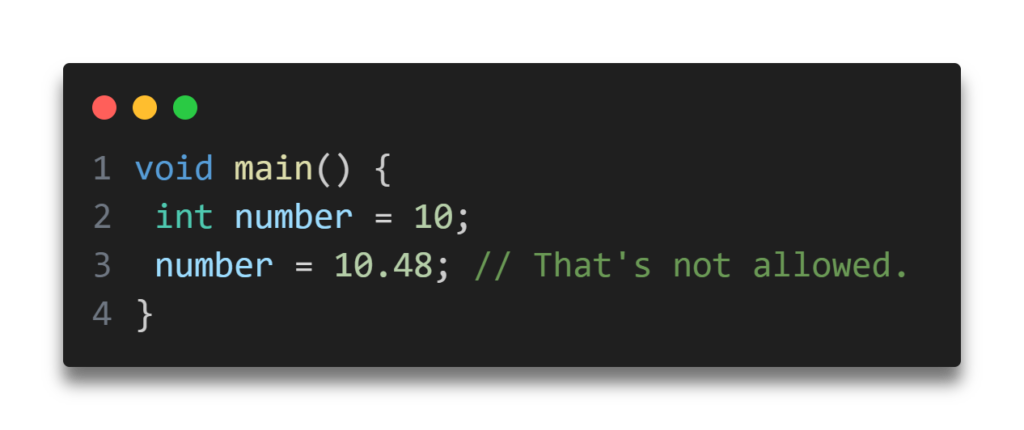
10.48 is a double, but you already defined number as an int. No changes allowed!
Dart’s type safety will save you countless hours when coding since the compiler will tell you immediately whenever you try to give a variable the wrong type. This prevents you from having to chase down bugs after you experience runtime crashes.
Of course, sometimes it’s useful to be able to assign related types to the same variable. That’s still possible. For example you could solve the problem above, where you want number to store both an integer and floating-point value, like so:
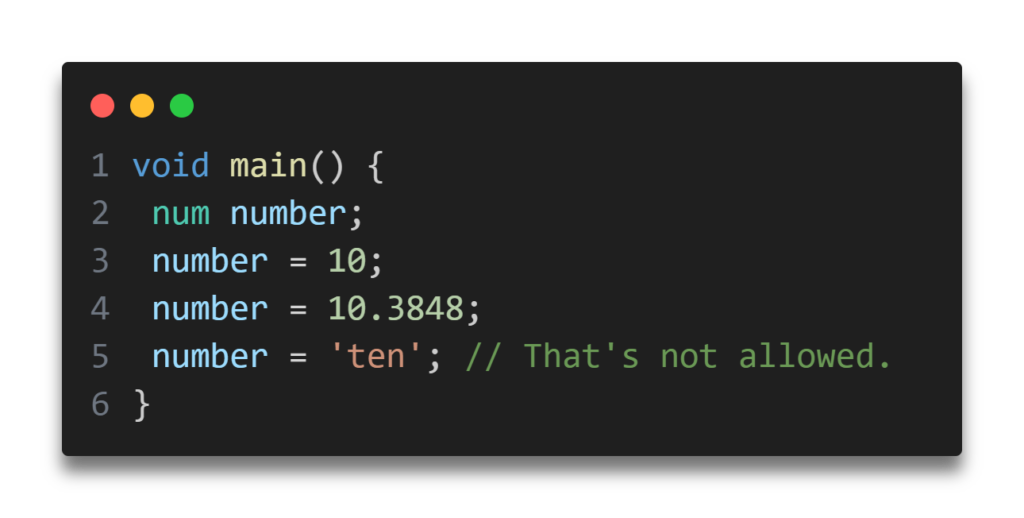
The num type can be either an int or a double, so the first two assignment work. However, the string value ‘ten’ is of a different type, so the compiler complains.
Now, if you like living dangerously, you can throw safety to the wind and use the dynamic type. This lets you assign any data type you like to your variable, and the compiler won’t warn you about anything.
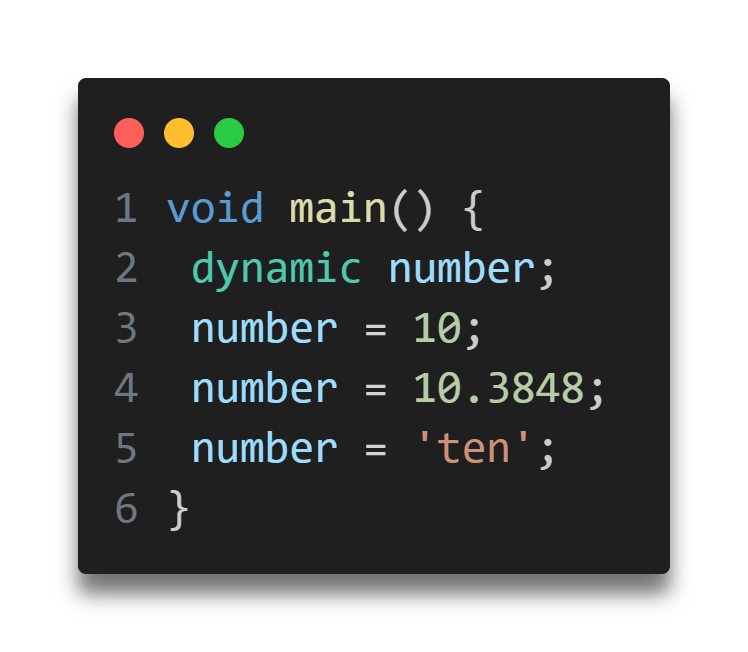
But, honestly, don’t do that. Friends don’t let friends use dynamic. Your life is too valuable for that.
Type inference in Dart
Dart is smart in a lot of ways. You don’t even have to tell it the type of a variable, and Dart can still figure out what you mean. The var keyword says to Dart, “Use whatever type is appropriate.“
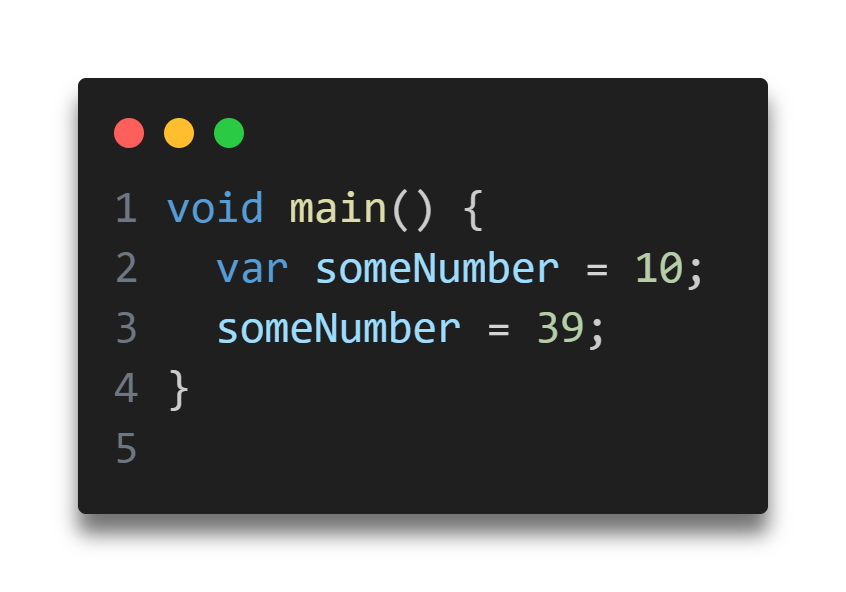
There’s no need to tell Dart that 10 is an integer. Dart infers the type and make someNumber an int. Type safety still applies, though:
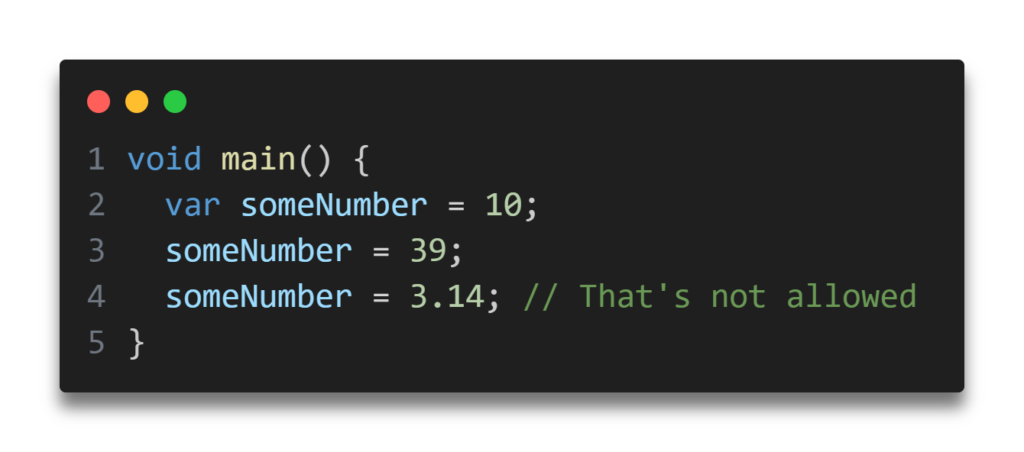
Trying to set someNumber to a double will result in an error. Your program won’t even compile. Quick catch; time saved. Thanks, Dart!
Constant in Dart
Dart has two different types of “variables” whose values never change. They are declared with the const and final keywords, and the following sections will show the difference between the two.
const constants
Variables whose value you can change are knows as mutable data.
Mutable certainly have their place in programs, but can also present problems. It’s easy to lose track of all the places in your code that can change the value of a particular variables.
For that reason, you should use constants rather than variables whenever possible. These unchangeable variables are know as immutable data.
to create a constant in Dart, use the const keyword:
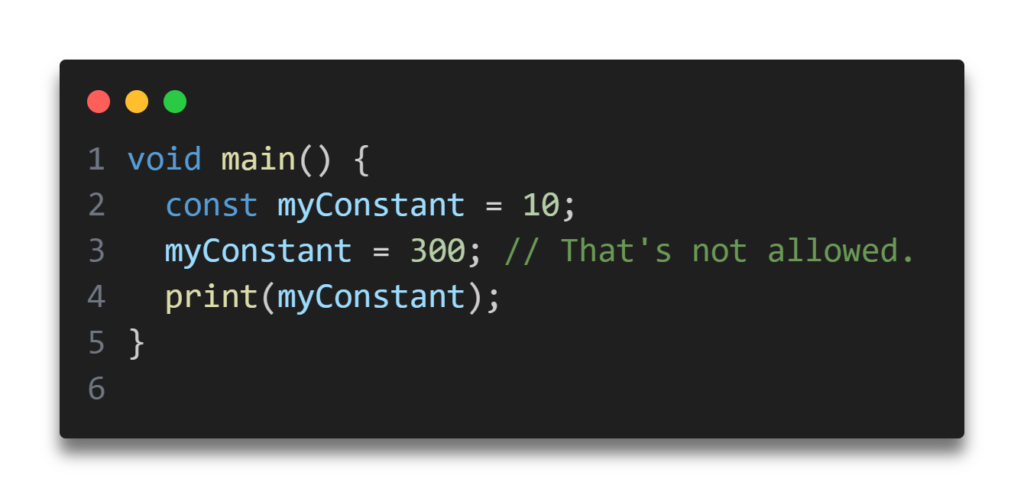
Just as with var, Dart uses type inference to determine that myConstant is an int.
Once you’ve declared a constant, you can’t change its data.
This code produces an error:

If you think “constant variable” sounds a little oxymoronic, just remember that it’s in good company: virtual reality, advanced BASIC, readable Perl and internet security.
final constants
Often, you know you’ll want a constant in your program, but you don’t know what its value is at compile time. You’ll only know the value after the program starts running.
This kind of constant is known as a runtime constant.
In Dart const is only used for compile-time constants; that is, for values that can be determined by the compiler before the program ever starts running.
If your can’t create a const variable because you don’t know its value at compile time, then you must use final keyword to make it a runtime constant. There are many reasons you might not know a value until after your program is running. For example, you might need to fetch a value from the server, or query the device settings, or ask a suer to input their age.
Here is another example of a runtime value:
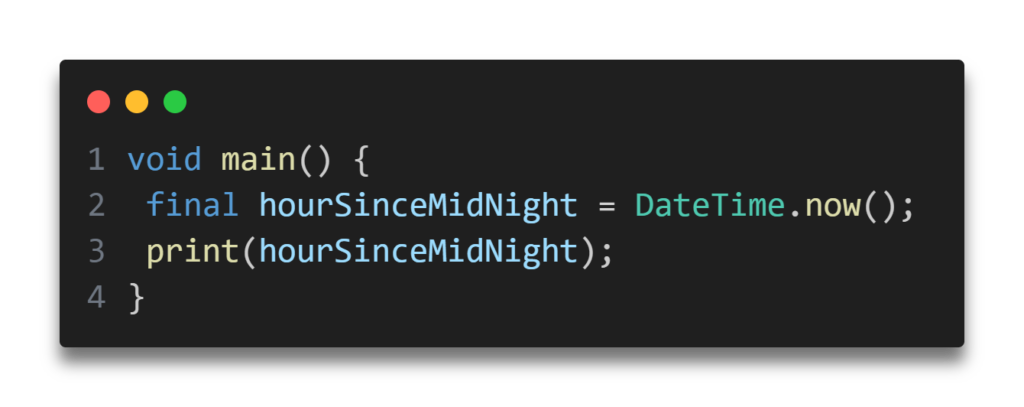
DateTime.now() is a Dart function that tells you the current data and time when the code is run. Adding hour to that tells you the number of hours that have passed since the beginning of the day. Since that code will produce a different results depending on the time of day, this is most definitely a runtime value. So to make hoursSinceMidnight a constant, you must use the final keyword instead of const.
If you try to change the final constant after it’s already been set:
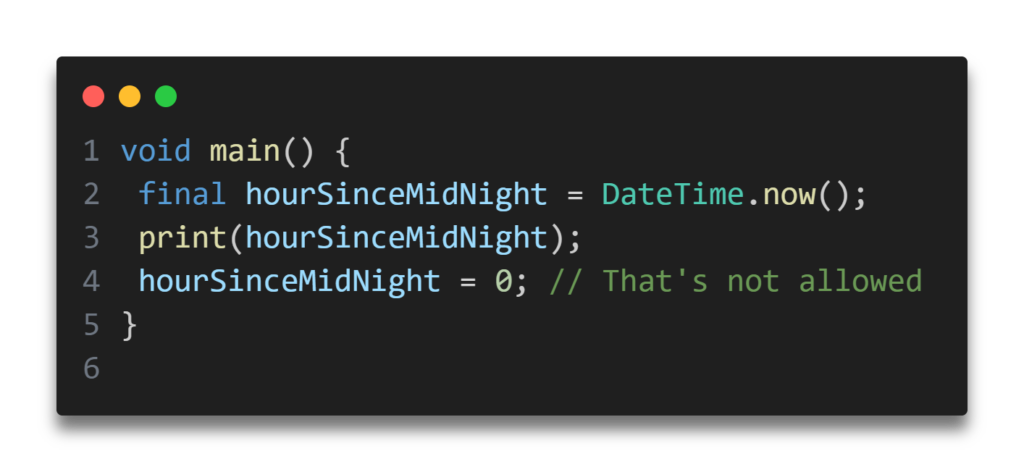
This will produce the following error:

You don’t actually need to worry too much about the difference between const and final constants. As a general rule, try const and final first, and if the compiler complains, then make it final. The benefit of using const is it gives the compiler the freedom to make internal optimizations to the code before compiling it.
No matter what kind of variables you have, though, you should give special consideration to what you call it.
Using meaningful names
Always try to choose meaningful names for your variables and constants. Good names act as documentation and make your code easy to read.
A good name specifically describes the role of a variable or constant. Here are some example of good names:
- personAge or person_Age
- numberOfPeople or number_Of_People
- gradePointAverage or grade_Point_Average
Often a bad name is simply not descriptive enough. Here are some examples of bad names:
- a
- temp
- average
The key is to ensure that you’ll understand what the variable or constant refers to when you read it again later. Don’t make the mistake of thinking you have an infallible memory!
It’s common in computer programming to look back at your own code as early as a day or two later and have forgotten what it does. Make it easier for yourself by giving your variables and constants intuiti8ve, precise names.
Also, note how the names above are written. In Dart, it’s standard to use lowerCamelCase for variable and constant names. Follow these rules to properly case your names:
- Start with a lowercase letter.
- If the name is made up of multiple words, join them together and start every word after the first one with an uppercase letter.
- Treat abbreviations like words (for example, sourceUrl and urlDescription).
Data Types in Dart
Life is full of variety, and that variety expresses itself in different types. What type is toothpaste do you use? Colgate? Spearmint? What’s your blood type? A? B? O+? What type of ice cream do you like? Vanilla? Strawberry?
Having names for all of these different things helps you talk intelligently about them. It also helps you to recognize when something is out of place. After all, no one brushes their teeth with parline pecan fudge swirl. Though it does sound kind of nice.
Programming types are just as useful as real life types. They help you to categorize all the different kinds of data you use in your code.
In Dart, a type is way to tell the compiler how you plan to use some data. By this point in this article, you’ve already seen the following types:
- int
- double
- num
- dynamic
- String
The last one in that list, String, is the type is the used for text like ‘Hello, Dart!’.
Just as you don’t brush your teeth with ice cream, Dart types keep you form trying to do silly things like dividing text or removing whitespace from a number.
Annotating variables explicitly
It’s fine to always explicitly add the type annotation when you declare a variable. This means writing the data type before the variable name.
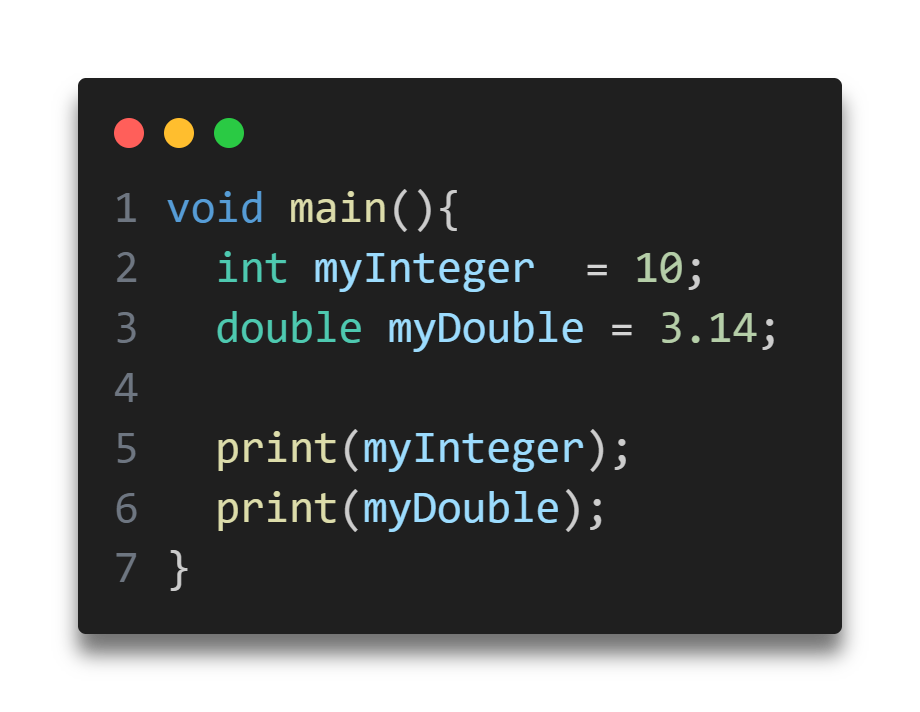
You annotated the first variable with int and the second with double.
Creating constant variables
Declaring variables the way you did above makes them mutable. If you want to make them immutable, but still keep the type annotation, you can add const or final in front.
These forms of declaration are fine with cosnt:
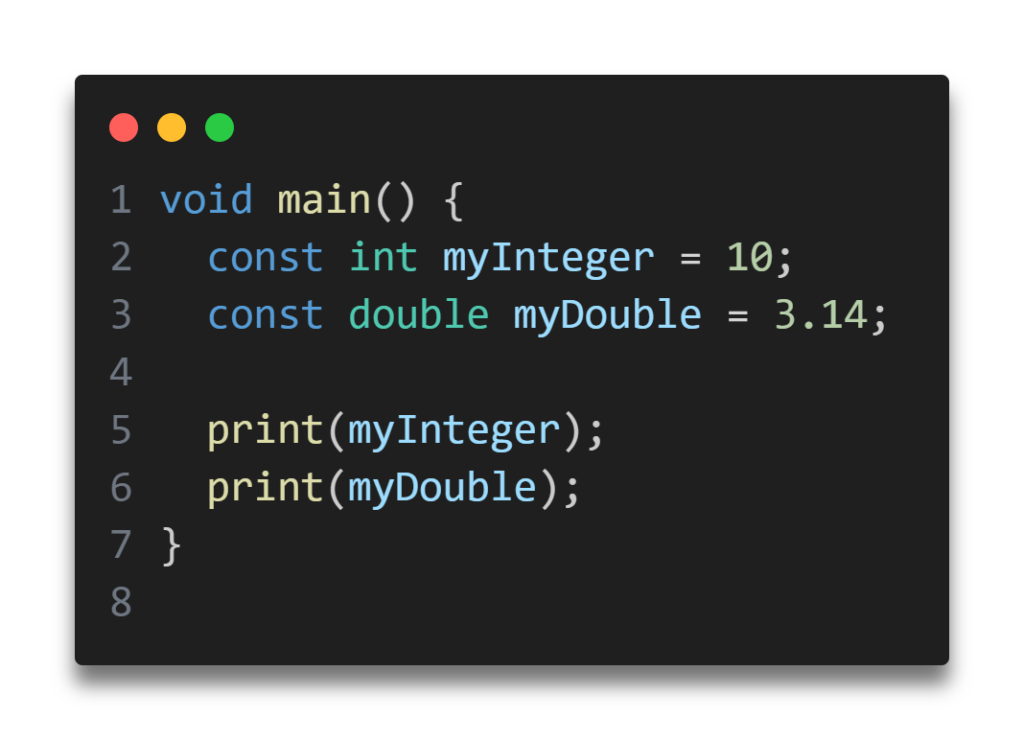
They’re also fine with final:
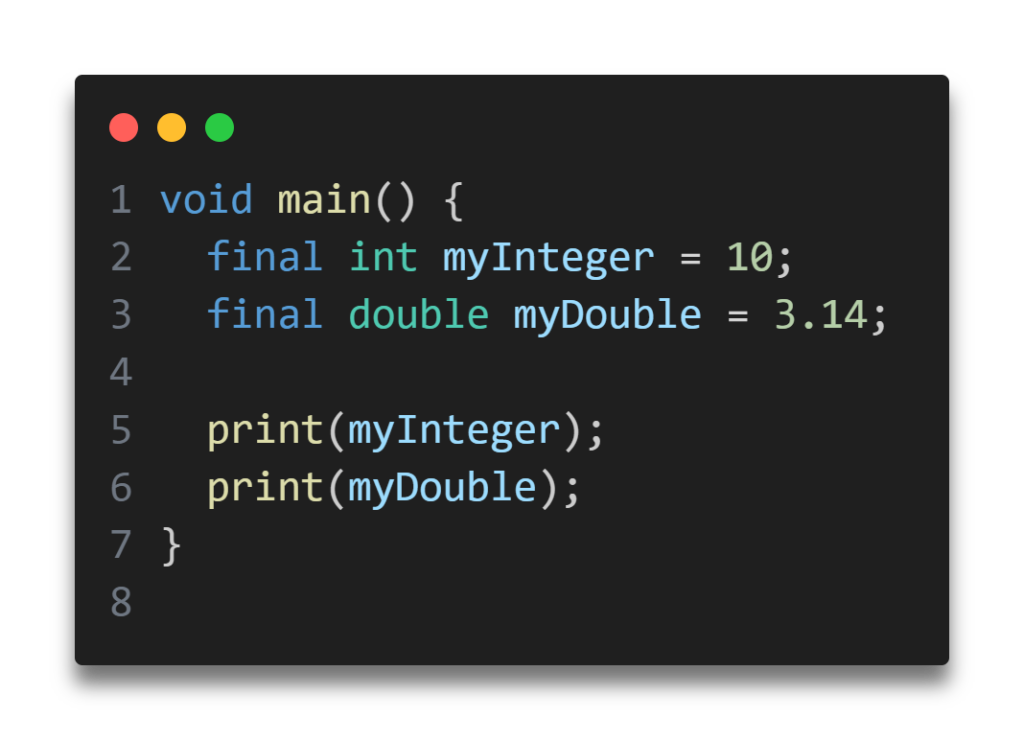
Note: Mutable data is convenient to work with because you can change it any time you like. However, many experienced software engineers have come to appreciate the benefits of immutable data. When a value is immutable, that means you can trust that no one will change that value after you create it. Limiting you data is this way prevents many hard-to-find bugs from creeping in, and also makes the program easier to reason about and to test.
Built-in types in Dart
The Dart language has special support for the following:
Numbers (int, double)
Dart numbers come in two flavors:
- int:
- Integer values no larger than 64 bits, depending on the platform. On native platforms, values can be from -263 to 263 – 1.
- int represents integer values (whole numbers) without any decimal places, like 10, -5, 1000.
- double
- 64-bit (double-precision) floating-point numbers, as specified by the IEEE 754 standard.
The IEEE 754 standard is widely adopted specification for representing and manipulating floating-point numbers in computer systems. It was established by the Institute of Electrical and Electronics Engineers (IEEE) in 1985, with revisions over the years, to ensure consistency and precisions in how computers handle decimal numbers, especially for scientific and engineering calculations. - double represents floating-point numbers, which are numbers with decimal points, like 3.14, 0.001, or -12.5.
- 64-bit (double-precision) floating-point numbers, as specified by the IEEE 754 standard.
Since both int and double are subclasses of num, they inherit the behavior and methods of the num class. This allows you to treat both integers and floating-point numbers as numeric values and perform operations that apply to any numeric type, such as addition, subtraction, multiplication, etc.
Why is this useful?
The num class allows flexibility when working with numbers because you can define a variable of type num, and that variable can hold either an int or a double. This makes the type system more convenient when you’re unsure whether a value will be an integer or a floating-point number.
Strings
Numbers are essential in programming, but they aren’t the only type of data you need to work with in your apps. Text is also a common data type, representing things such as people’s names, their addresses, or even the complete text of a book. All of these are examples of text that an app might have to handle.
Most computer programming languages store text in a data type called a string.
How computers represent strings
Computer thinks of strings as a collection of individual characters. Numbers are the langauge of CPUs, and all code, in every programming language, can be reduced to raw numbers. Strings are no different.
That may sound very strange. How can characters be numbers? At its base, a computer needs to be able to translate a character into the computer’s own langauge, and it does so by assigning each character a different number. This forms a two-way mapping from character to number that’s called a character set.
When you press a character key on your keyboard, you are actually communicating the number of the character to the computer. You computer converts that number into a picture of the character and finally, presents that picture to you.
Unicode
In isolation, computer is free to choose whatever character set mapping it likes. If the computer wants the letter a to equal the number 10, then so be it. But when computers start talking to each other, they need to use a common character set.
If two computers used different character then when one computer transferred a string to the other, they would end up thinking the string contained different characters.
There have been several standards over the years, but the most modern standard is Unicode. It defines the character set mapping that almost all computers use today.
Note: You can read more about Unicode at its official website, unicode.org.
As an example, consider the word cafe. The Unicode standard tells us that the letters of this word should be mapped to numbers like so:
c | a | f | e |
99 | 97 | 102 | 101 |
The number associated with each character is called a code point. So in the example above, c used code point 99, a uses code point 97, and so on.
Of course, Unicode is not just for the simple Latin characters used in English, such as c, a, f and e. It also lets you map characters from languages around the world. The word cafe, as you’re probably aware, is derived from French, in which it’s written as café.
Unicode maps these characters like so:
c | a | f | é |
99 | 97 | 102 | 233 |
Working with strings in Dart
Dart like any good programming langauge, can work directly with strings. It does so through the String data type.
Strings and characters
Let’s see this code:
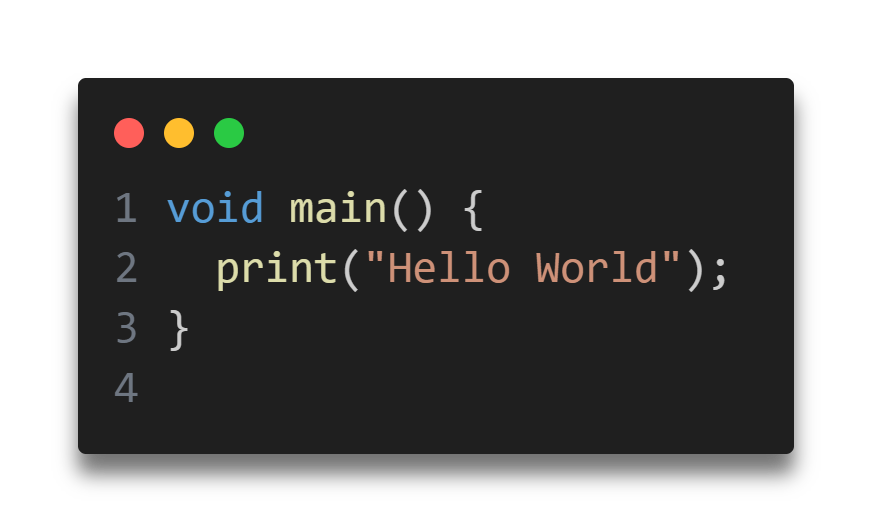
You can extract the same string as a named variable:
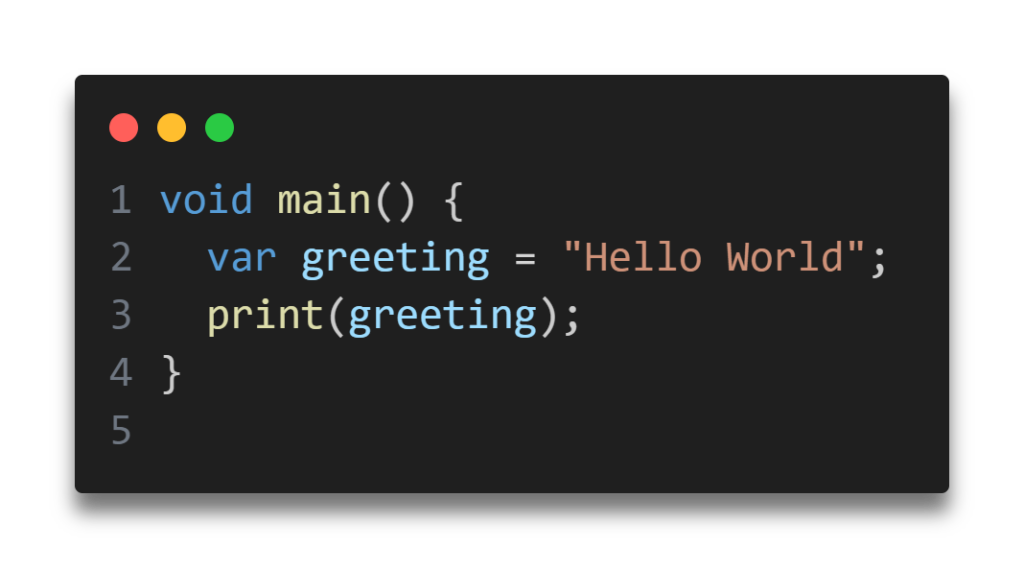
The right-hand side of this expression is know as a string literal. Due to type inference, Dart knows that greeting is of type String. Since you used the var keyword, you’re allowed to reassign the value of greeting as long as the new value is still a string.
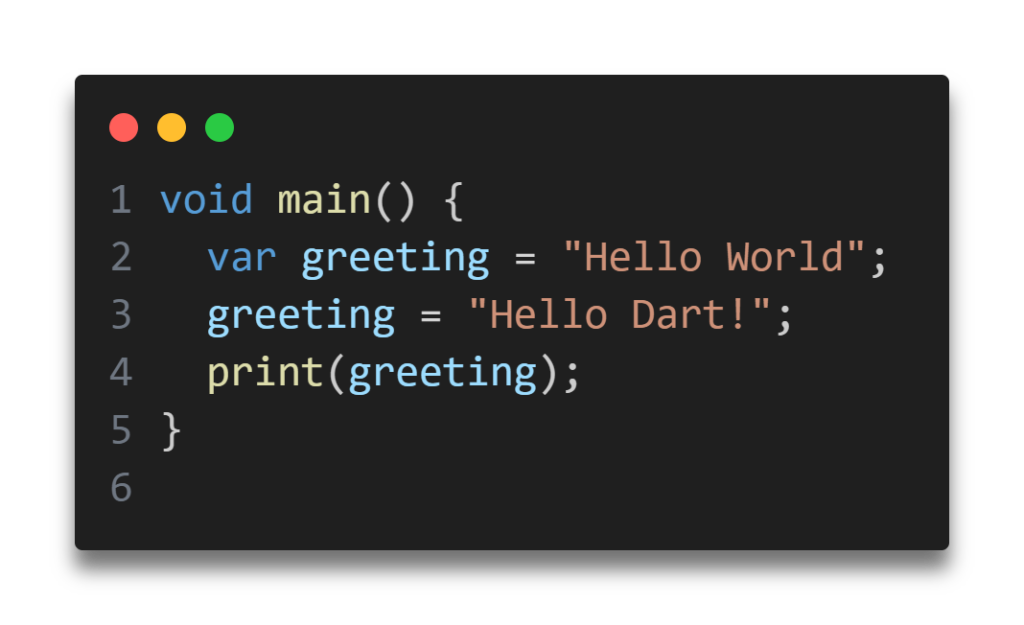
Even through you changed the value of greeting here, you didn’t modify the string itself. That’s because strings are immutable in Dart. It’s not like you replaced Dart in the first string with Dart!. No, you completely discarded the string “Hello, World” and replaced it with a whole new string whose value was “Hello Dart!”.
Booleans
A Boolean in dart is a data type that can hold only one of two possible values: true or false. These values are used to represent logical conditions and are often used in decision-making (like if statements) or to control the flow of a program.
- bool Data Type: In Dart, the
bool
type is used to store Boolean values. It can only hold either true of false. - Comparison Operations: Booleans are commonly the result of comparison operations like checking if one value is greater than or equal to another.
- Logical Operations: You can use Booleans with logical operators like AND (&&), OR (||), and NOT(!) to combine or invert conditions.
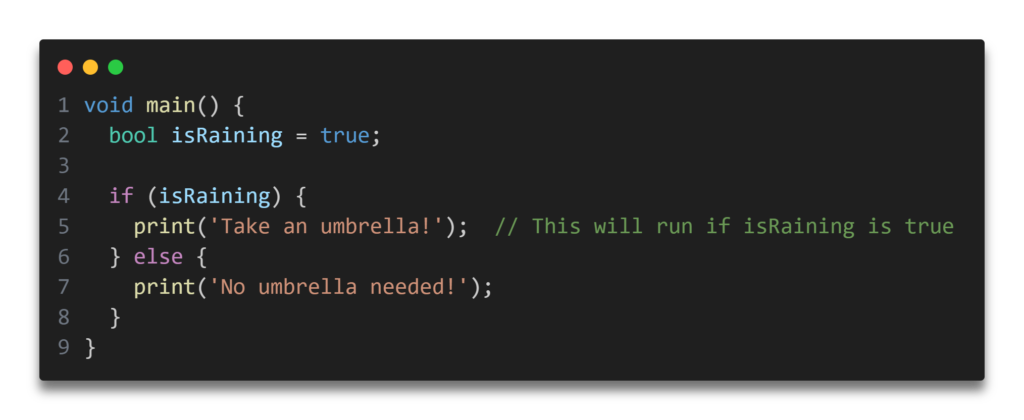
Lists in Dart
Whenever you have a very large collection of objects of a single type that have an ordering associated with them, you’ll likely want to use a list as the data structure for ordering the objects. Lists in Dart are similar to arrays in other languages.
The image below represents a list with six elements. Lists are zero-based, so the first element is at index 0. The value of the first element is cake, the value of the second element is pie, and so on until the last element at index 5, which is cookie.
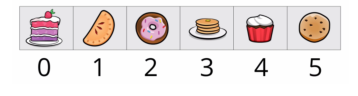
The order of a list matters. Pie is after cake, but before donut. If you loop through the list multiple times, you can be sure elements will stat in the same location and order.
Basic list operations
You’ll start by learning how to create lists and modify elements.
Creating a list
You car create a list by specifying the initial elements of the list within square brackets. This is called a list literal.
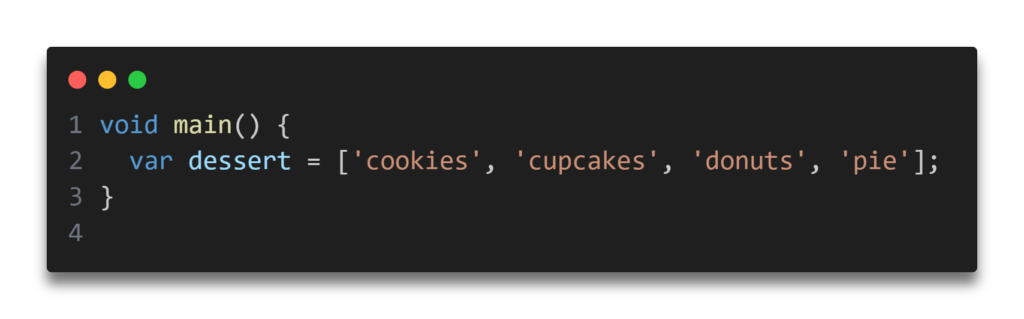
Since all of the elements in this list are strings Dart infers this to be a list of Strings types.
You can reassign desserts (but why would one ever want to reassign desserts?) with an empty list like so:
Dart still knows that desserts is a list of strings. However, if you were to initialize a new empty list like this:
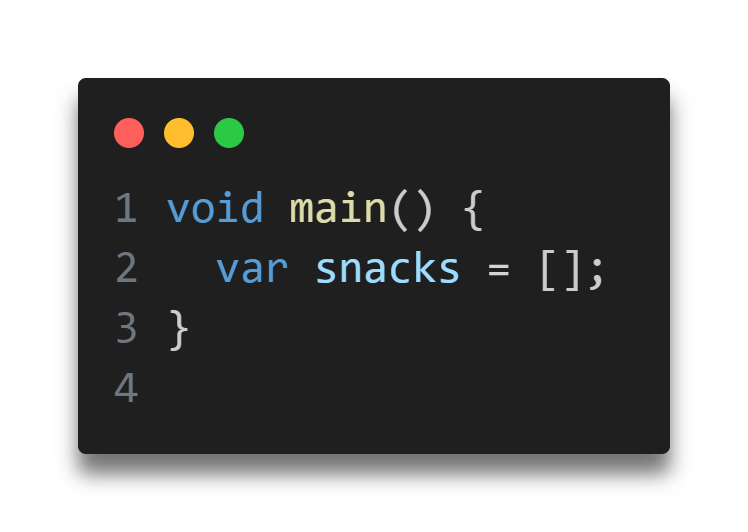
Dart wouldn’t have enough information to know what kind of objects the list should hold. In this case, Dart simply infers it to be a list of dynamic. This causes you to lose type safety, which you don’t want. If you’re starting with an empty list, you should specify the type like so:
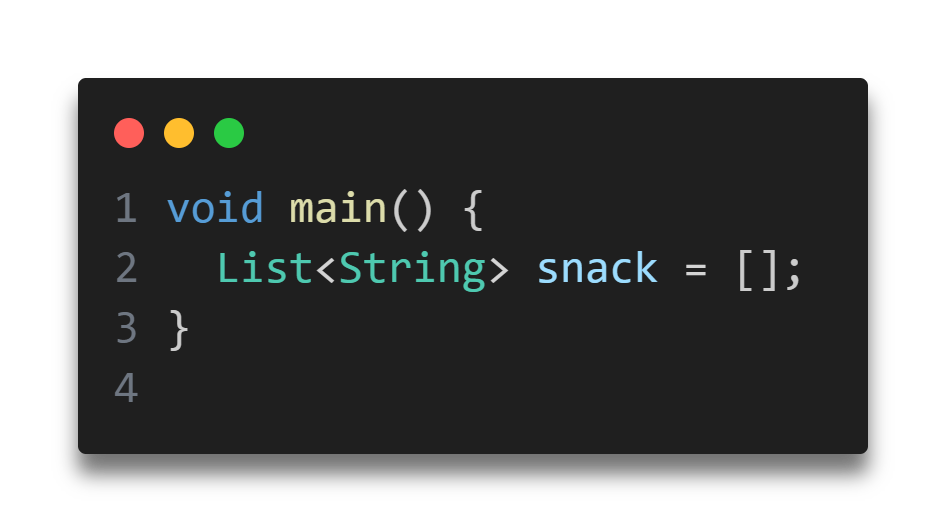
There are a couple of details to note here:
- The angle brackets <> here are the notation for generic types in Dart. A generic list means you can have a list of anything; You just put the type you want inside the angle brackets. In this case, you have a list of strings, but you could replace String with any other type.
For example, List<int> would make a list of integers, List<bool> would make a list of Booleans, and List<Grievance> would make a list of grievances — but you’d have to define that type yourself since Dart doesn’t come with any by default.
A slightly nicer syntax for creating an empty list is to use var or final and move the generic type to the right:
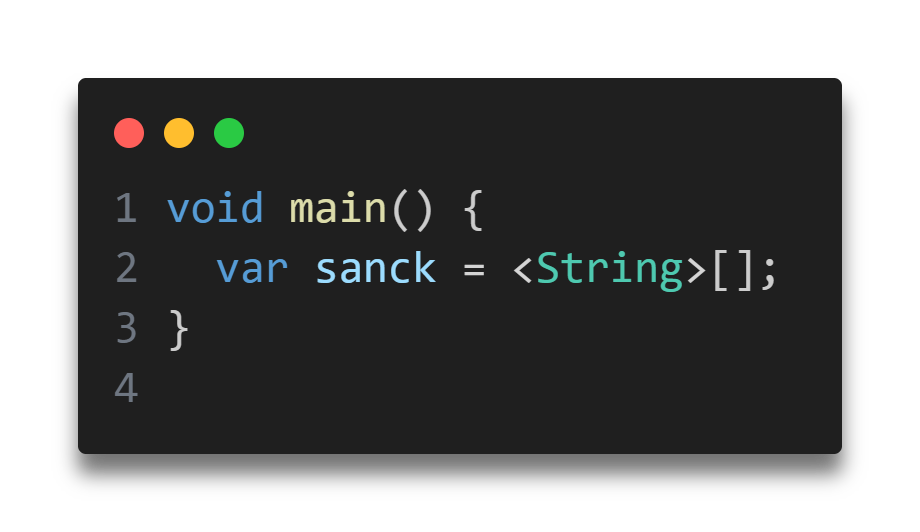
Dart still has all the information it needs to know this is an empty list of type String.
Accessing elements
To access the elements of a list, you reference its index via subscript notation, where the index number goes within square brackets after the list name.
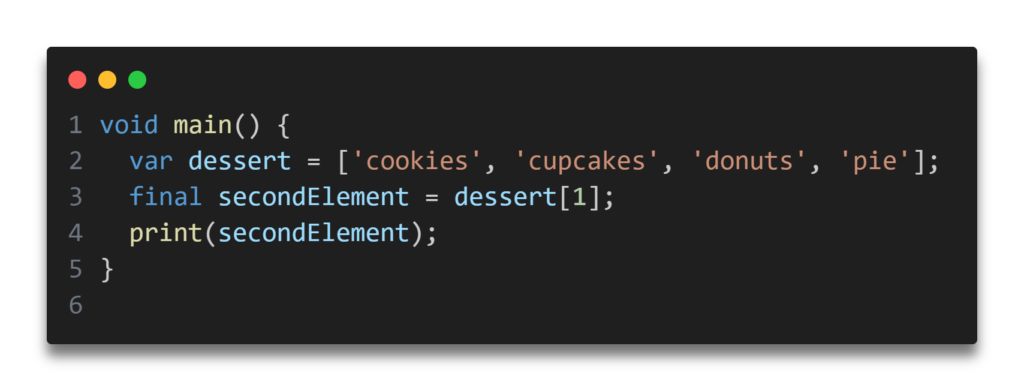
Don’t forget that lists are zero-based, so index 1 fetches the second element. Run that code and you’ll see cupcakes as expected.
If you know the value but don’t know the index, you can use indexOf method to look it up:
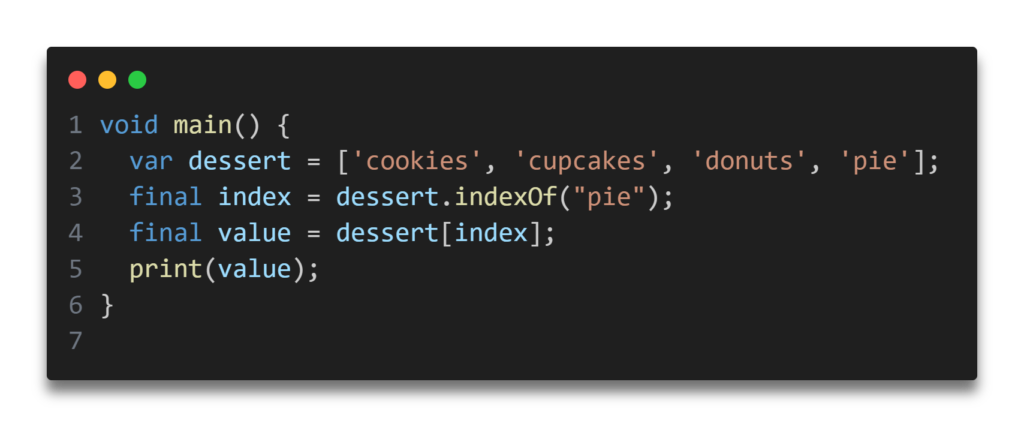
Since ‘pie’ is the fourth item in the zero-based list, index is 3 and value is pie.
Assigning values to list elements
Just as you access elements, you also assign values to specific elements using subscript notation:
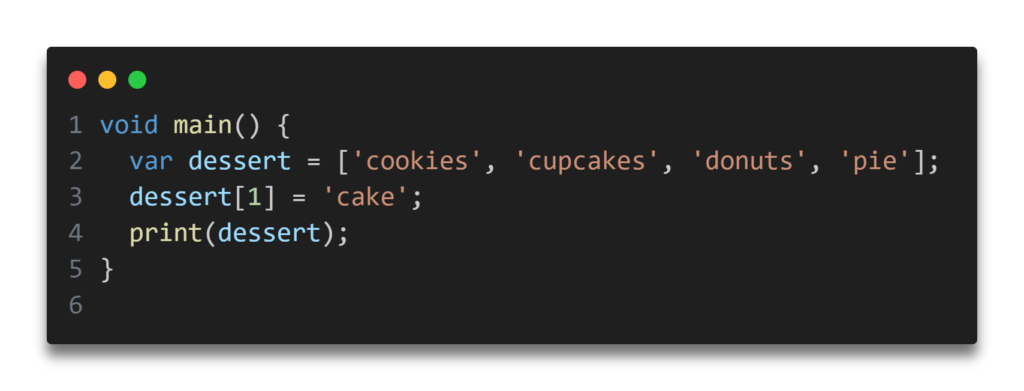
This changes the value at index 1 from cupcakes to cake.
Adding elements to a list
Lists are growable by default in Dart, so you can use the add method to add an element.
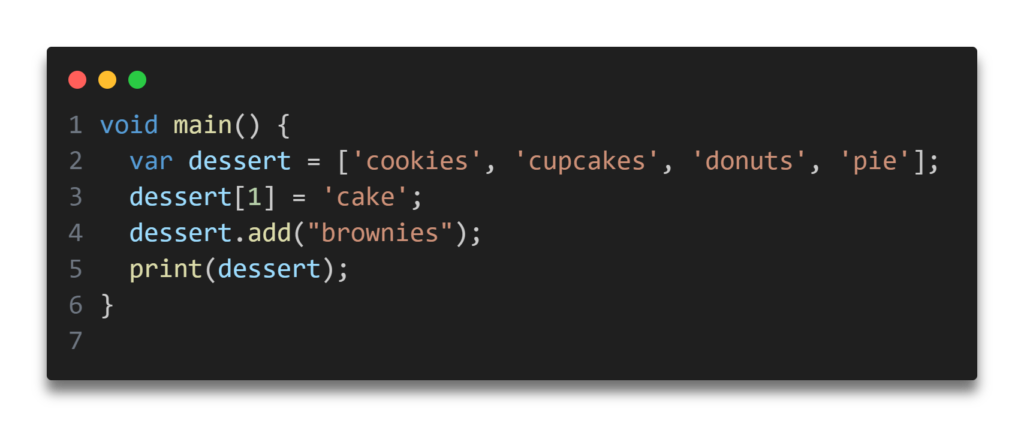
Run that and you’ll see:
[ cookies, cake, donuts, pie, brownies ]
Now desserts has five elements and the last one is brownies.
Removing elements from a list
You can remove elements using the remove method. So if you’d gotten a little hungry and eaten the cake, you’d write:
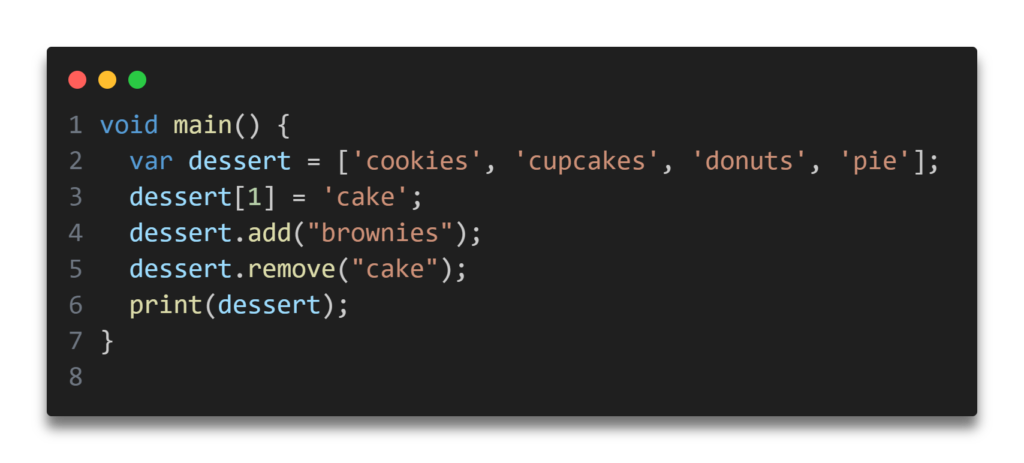
[cookies, dounts, pie, brownies]
No worries, there’s still plenty for a midnight snack tonight!
List properties
Collections such as List have a number of properties. To demonstrate them, use the following list of drinks.
Accessing first and last elements
You can access the first and last element in a list:
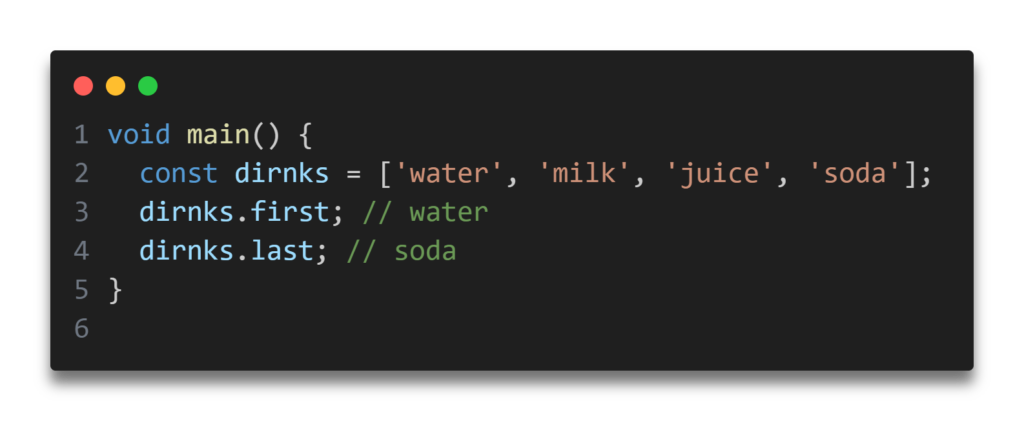
Checking if a list contains any elements
You can also check whether a list is empty or not empty.
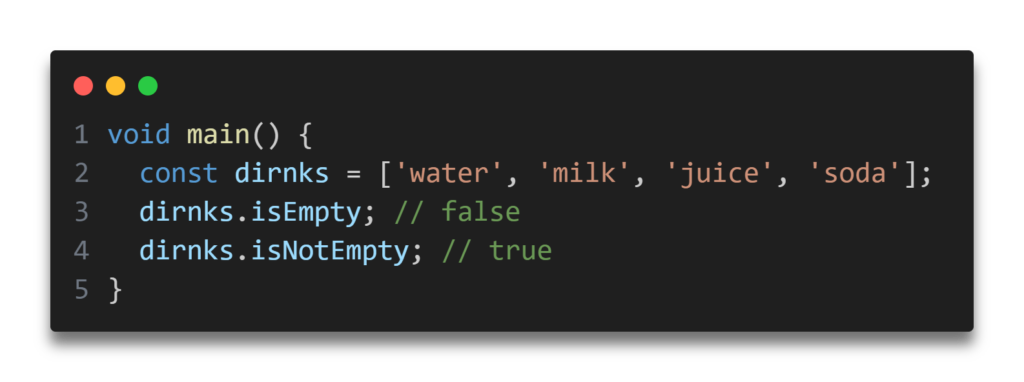
This is equivalent to the following:
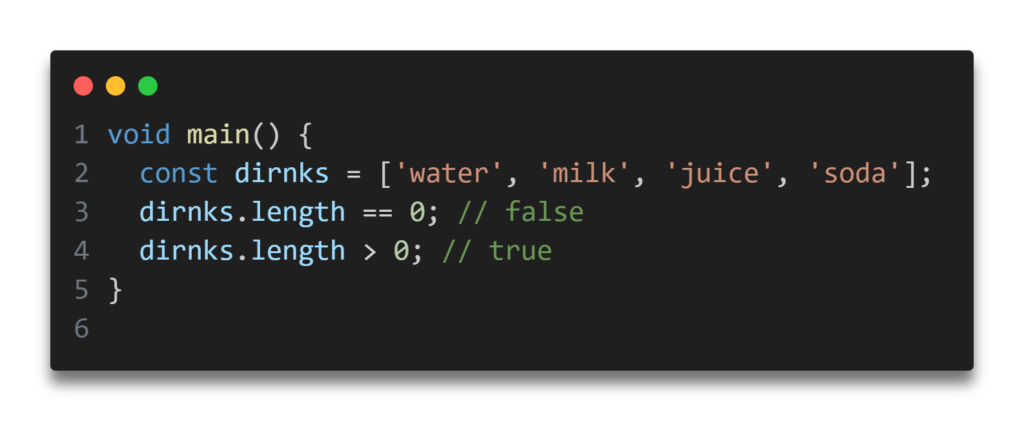
However, it’s more readable to use isEmpty an isNotEmpty.
Records
In Dart, records are a relatively new feature that allows you to group multiple values together creating a custom class or using a list or map.
Think of records as lightweight containers for grouping data. They car hold different types of values, but they don’t have the overhead or complexity of creating a full class.
Key Points about Records:
- Simple Structure:
- A record is a fixed collection of values (like a tuple in other languges).
- The values can be of different types.
- No Class Required:
- Unlike objects, you don’t need to define a class to group values. You can just create a record on the fly.
- Positional and Named Fields:
- You can use positional fields (by their index) or named fields (by a label you assign to each value).
- Immutable:
- Records are immutable, which means once you create them, you can’t change their values.
Syntax:
- A record with positional fields looks like this:
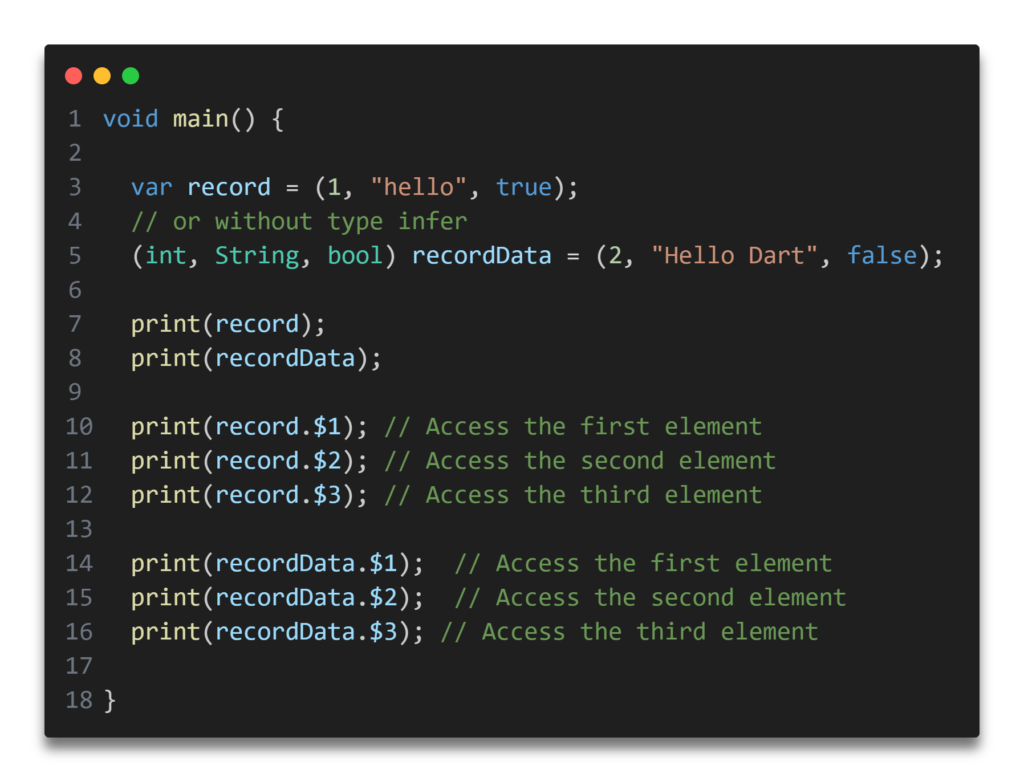
Sets
Sets are used to create a collection of unique elements. Sets in Dart are similar to their mathematical counterparts.
Duplicates are not allowed in a set, in contrast to list, which do allow duplicates.

You can also think of sets as a bag of elements with no particular ordering, unlike list, which do maintain a specific order. Because order doesn’t matter in a set, sets can be faster than lists, especially when dealing with large datasets.
Creating a set
You can create an empty set in Dart using the Set type annotation like so:
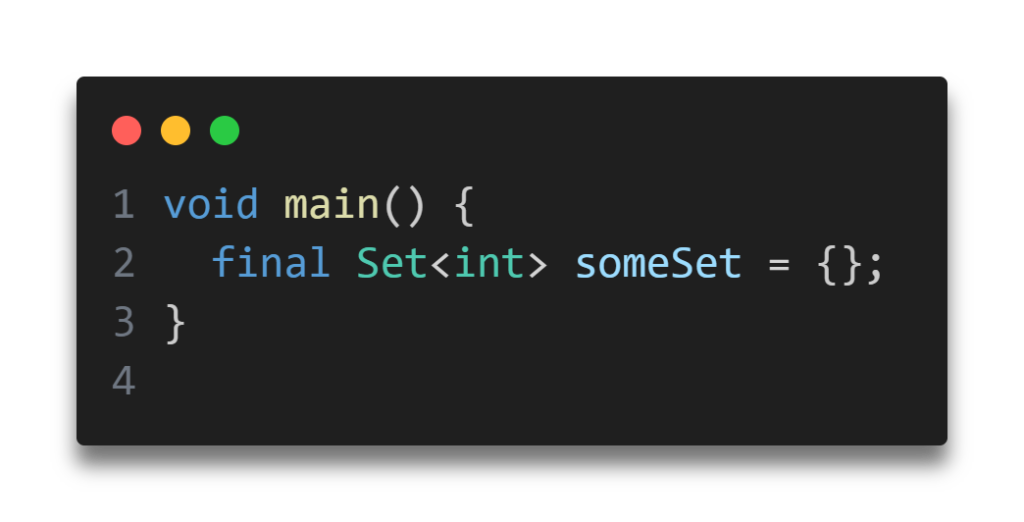
The generic syntax with int in angle brackets tells Dart that only integers are allowed in the set.
The curly braces are the same symbols used for sets in mathematics, so that should help you remember them.
Be sure to distinguish curly braces in this context from their use for defining scopes, though.
You can also use type inference with a set literal to let Dart determine the types of elements in the set.
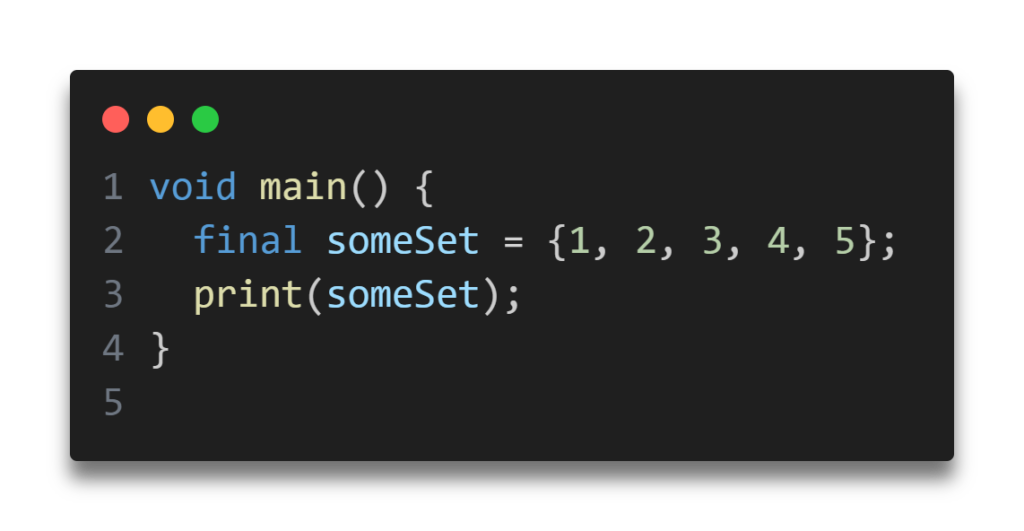
Operations on a set
Checking the contents
To see if set contains an item, you use the contains method, which returns a bool.
Add the following two lines and run the code again:
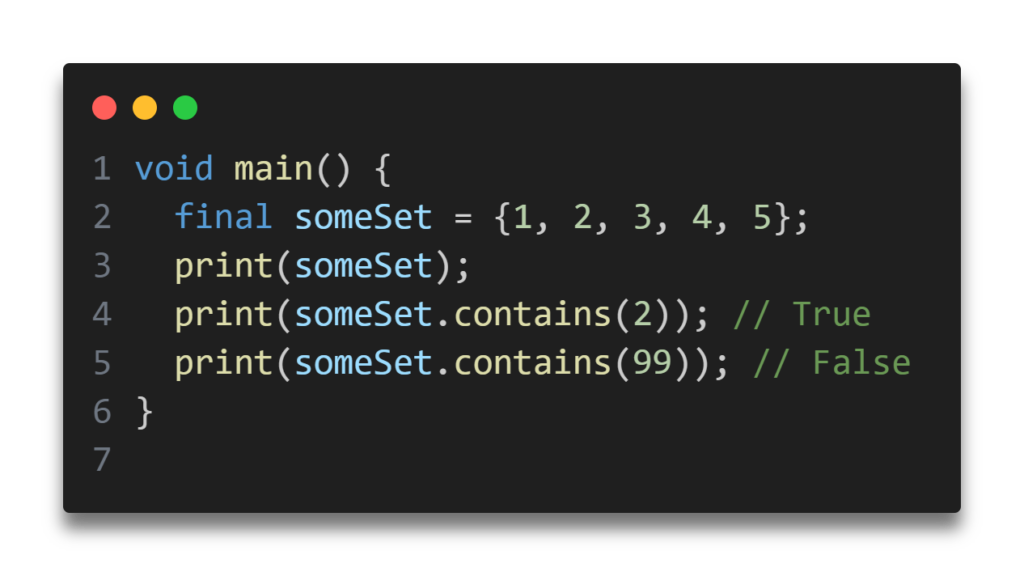
Since anotherSet does contains 1, the method returns true, while checking for 99 returns false.
Adding single elements
Like growable lists, you can add and remove elements in a set. To add an element, use the add method.
Run that to see the following set:
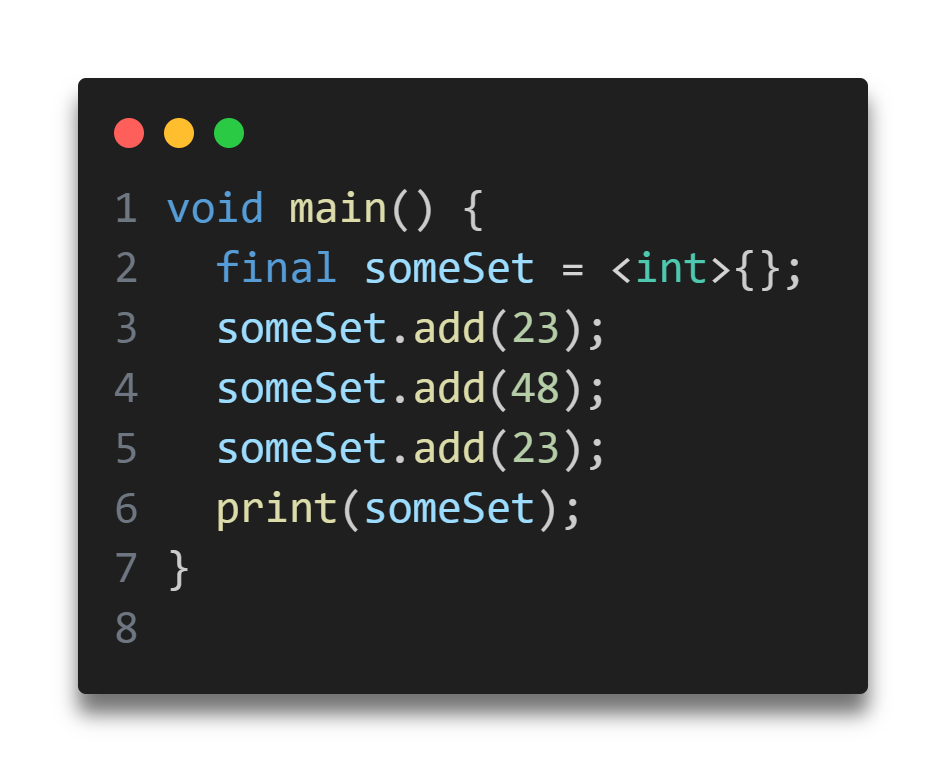
{ 23, 48, 31 }
you added 23 twice, but only one 23 shows up as expected.
Removing elements
You can also remove elements using the remove method.
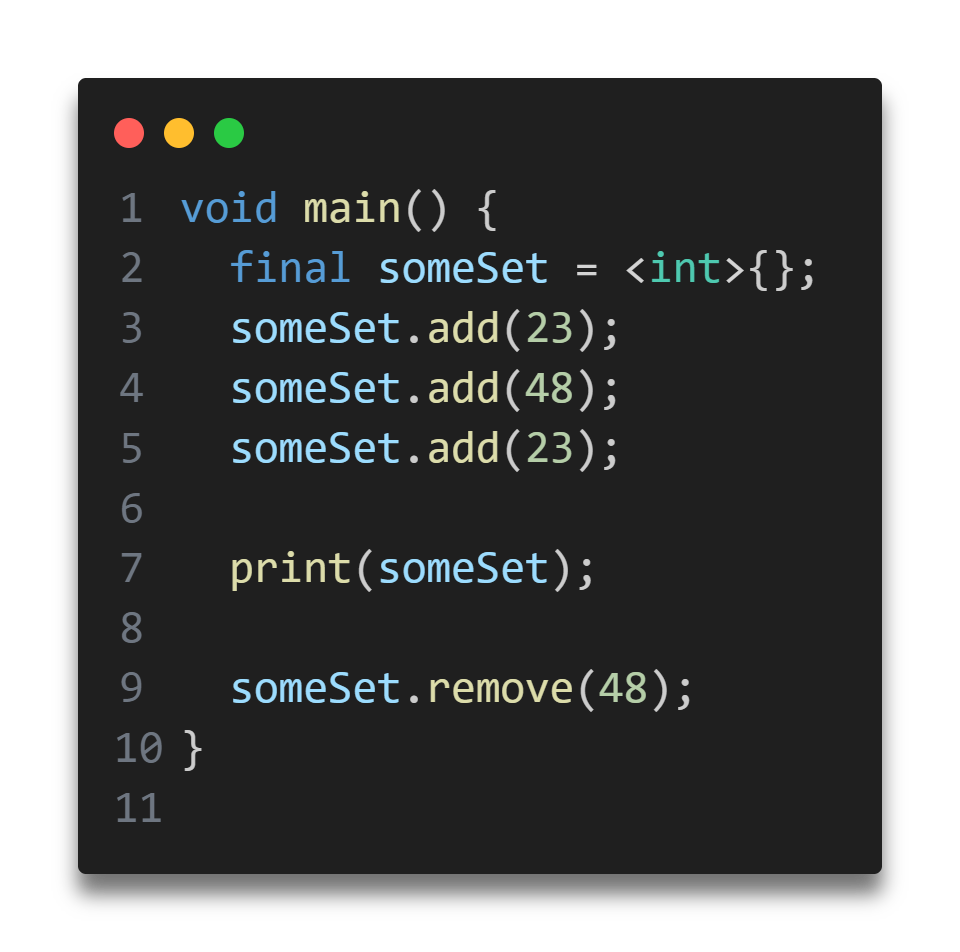
Adding multiple elements
You can use addAll to add elements from a list into a set.
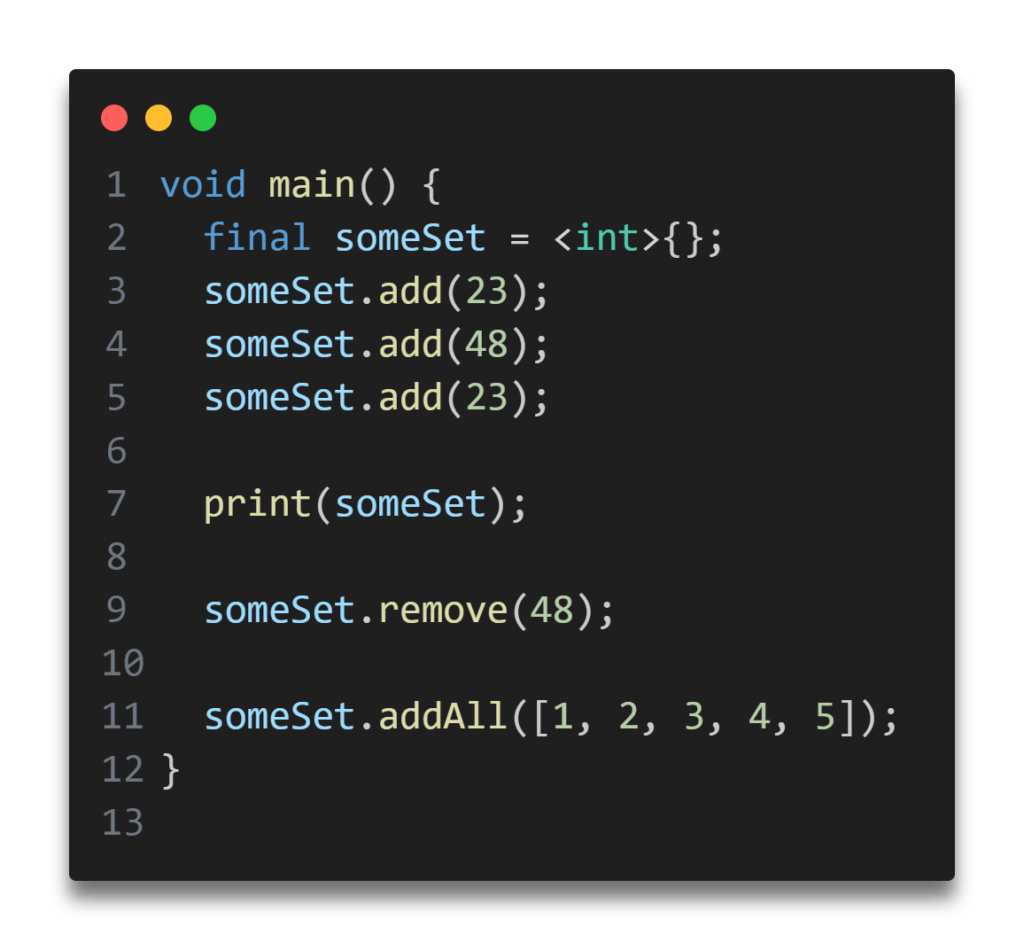
Maps
Maps in Dart are the data structure used to hold key-value pairs. They’re similar to HashMaps and Dictionaries in other languages.
If you’re not familiar with maps, though, you can think of them like a collection of variables that contain data. The key is the variable name and the value is the data that the variable holds. The way to find a particular value is to give the map the name of the key that is mapped to that value.
In the image below, the cake is mapped to 500 calories, and the donut is mapped to 150 calories. cake and donut are keys, while 500 and 150 are values.
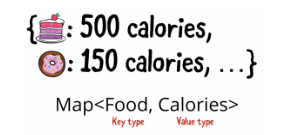
The key and value in each pair are separated by colons, and consecutive key-value pairs are separated by commas.
Creating an empty map
Like List and Set, Map is a generic type, but Map takes two type parameters: one for the key and one for the value. You can create an empty map variable using Map and specifying the type for both the key and value:
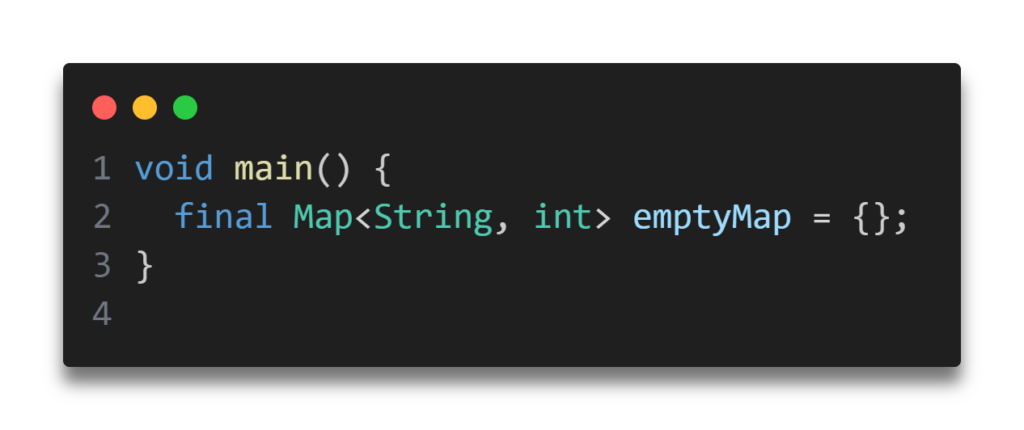
In this example, String is the type for the key, and int is the type for the value.
A slightly shorter way to do the same thing is move the generic types to the right-hand side:
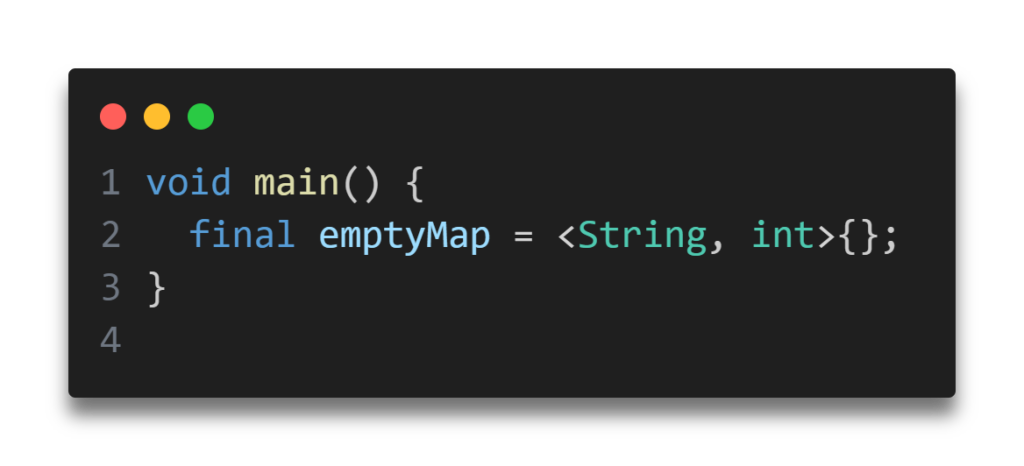
Notice that maps also use curly braces just as sets do. What do you think you’d get if you wrote this?
final emptySomething = {};
Is emptySomething a set, or is it a map?
Well, it turns out that map literals came before set literals in Dart’s history, so Dart infers the empty braces to be a Map of <dynamic, dynamic>; that is, the type of the key and value are both dynamic. If you want to set, and not a map, then you need to explicit.
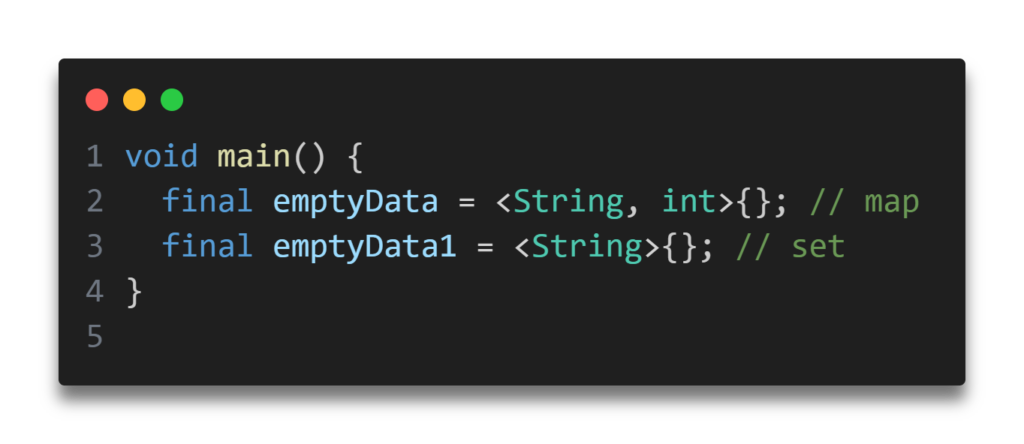
Initializing a Map with values
You can create a non-empty map variable using braces, where Dart infers the key and value types. Dart knows it’s a map because each element is a pair separated by a colon.
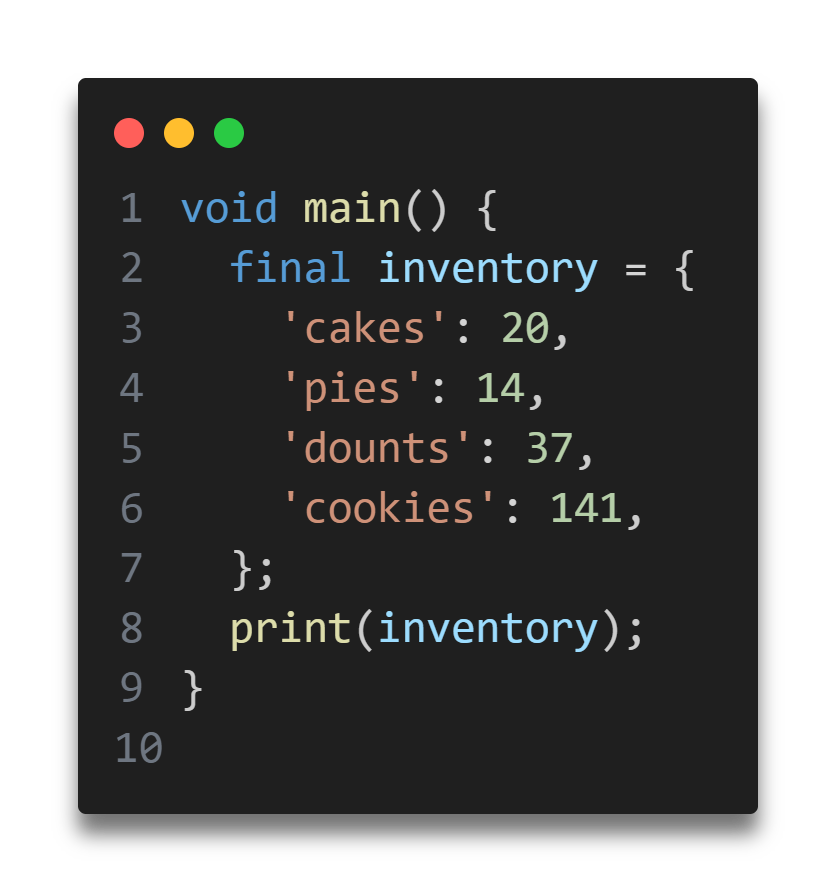
In this case, inventory is a map of String to int, from bakery item to quantity in stock.
The key doesn’t have to be a string. For example, here’s a map of int to String, from a digit to its English spelling:
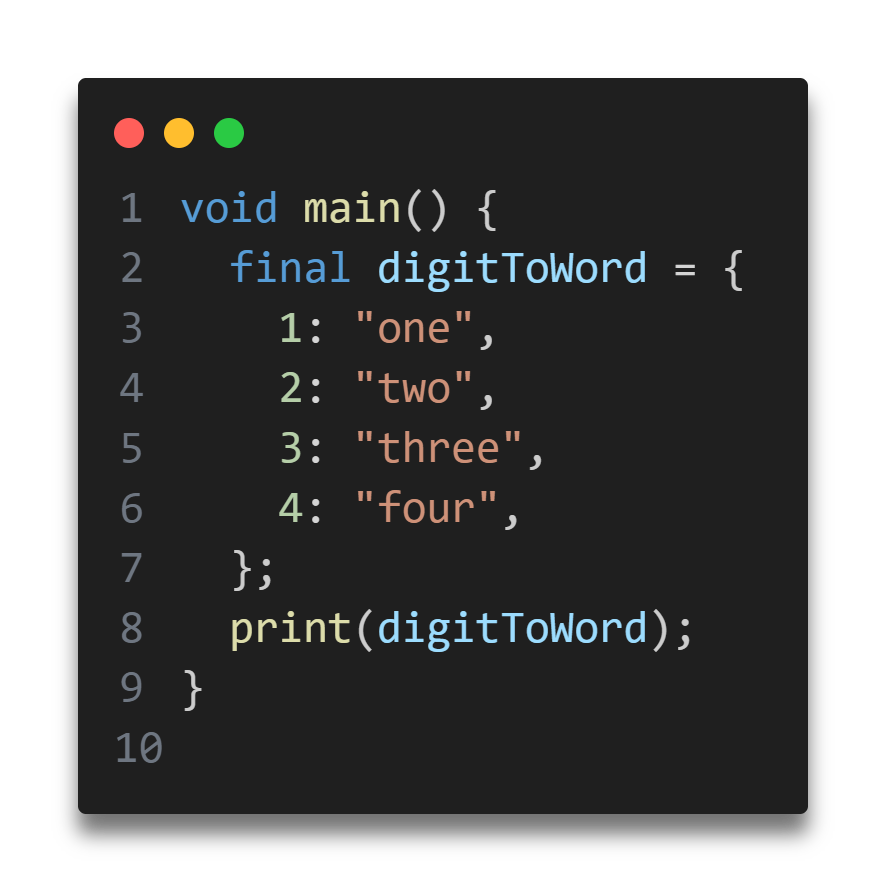
Unique keys
The keys of a map should be unique. A map like the following wouldn’t work:
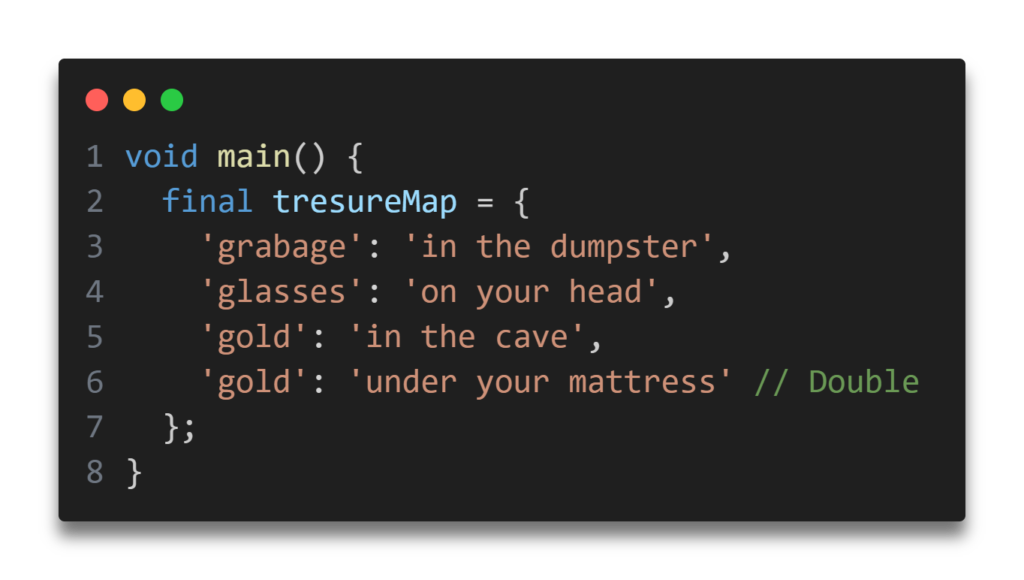
There are two keys named gold. How are you going to know where to look? You’re probably thinking, “Hey, it’s gold. I’ll just look both places.” If you really wanted to set it up like that, then you could map String to List:
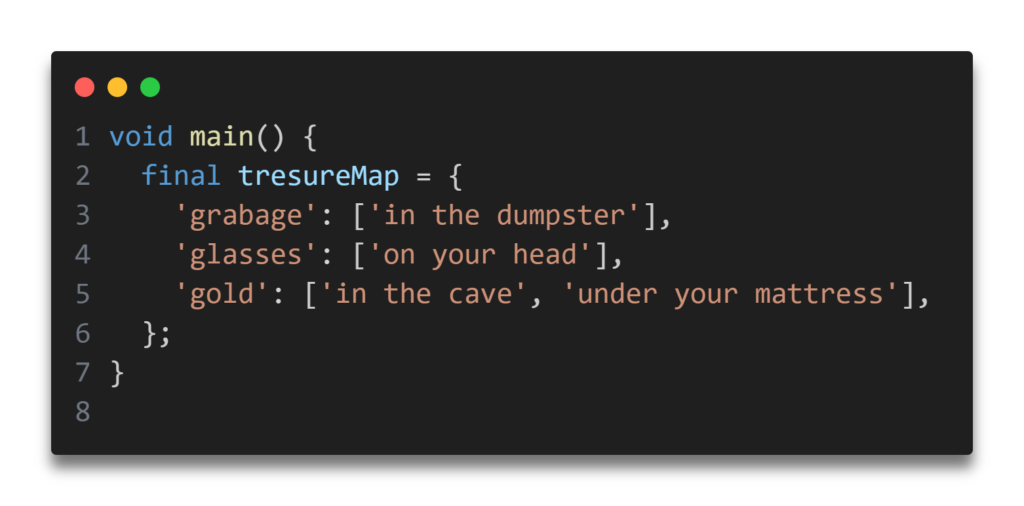
Now every key contains a list of items, but the keys themselves are unique.
Values don’t have that same restriction of being unique. This is fine:

Operations on a map
Interacting with a map to access, add, remove and update elements is very similar to what you’ve already seen.
Accessing elements from a map
You access individual elements from a map by using a subscript notation similar to lists, except for maps you use the key rather than an index.
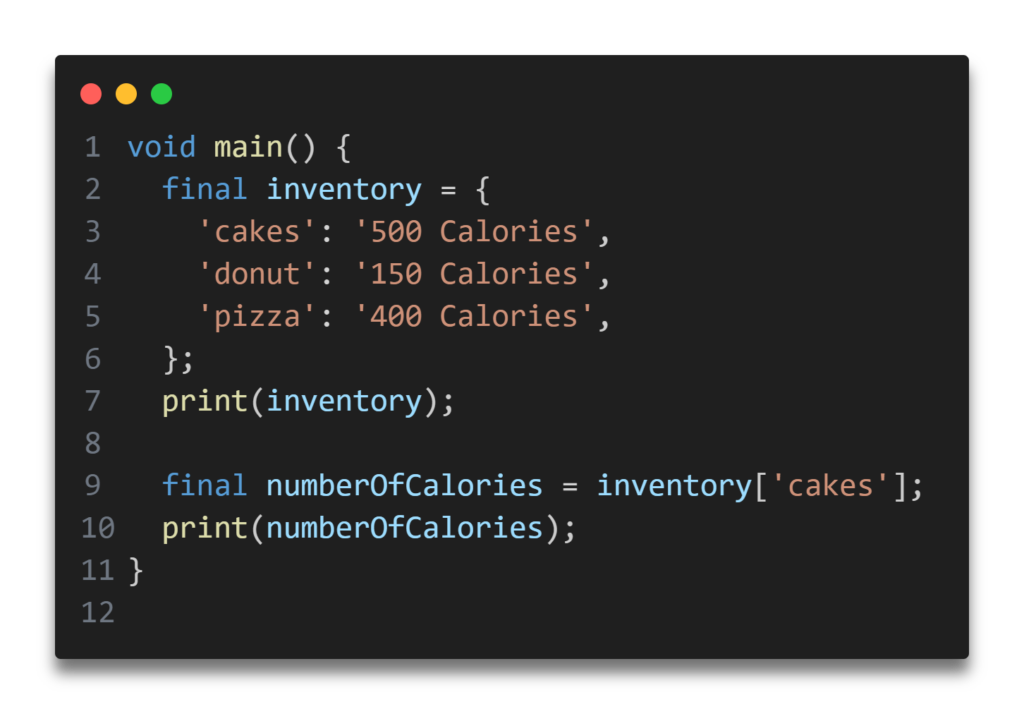
A map will return null if the key doesn’t exist. Because of this, accessing an element from a map always gives a nullable value.
Adding elements to a map
You can add new elements to a map simply by assigning to elements that are not yet in the map.
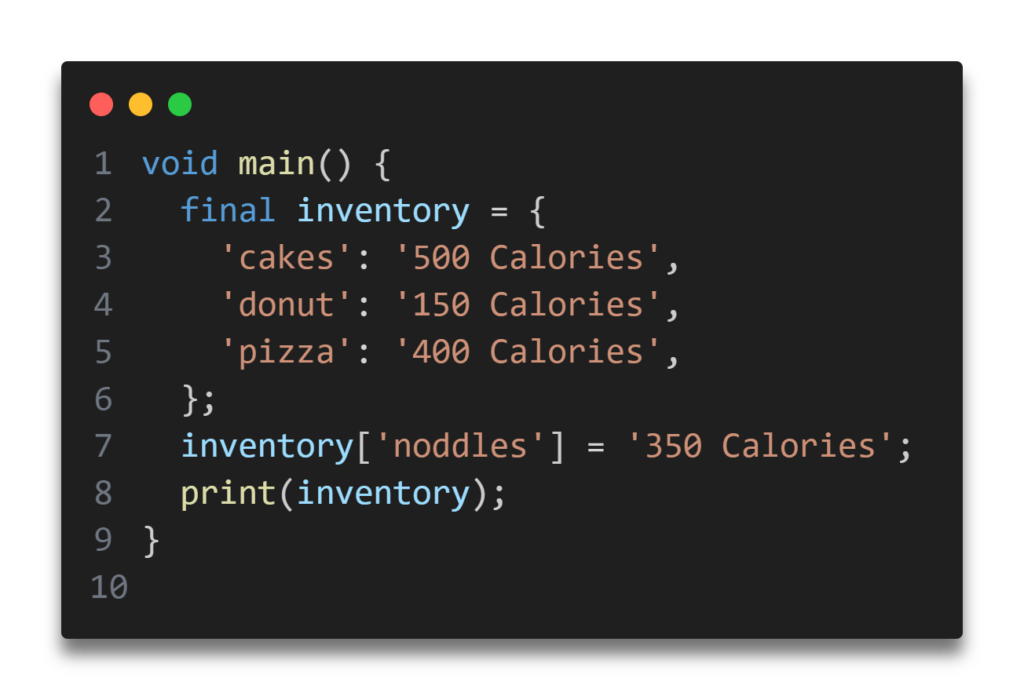
Updating an element
Remember that the keys of a map are unique, so if you assign a value to a key that already exists, you’ll overwrite the existing value.

Removing elements from a map
You can use remove to remove elements from a map by key.
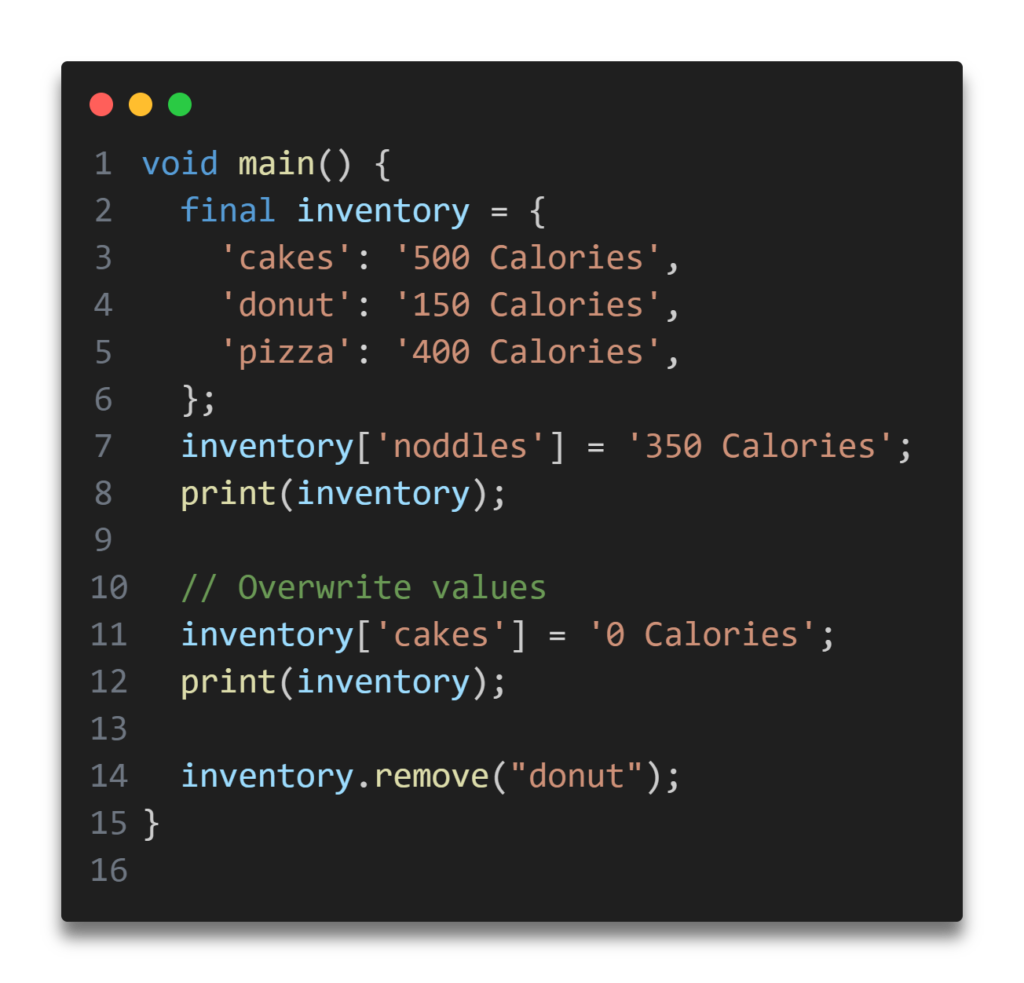
DONUT! Om nom nom nom nom nom.
No more donuts.
Map properties
Maps have properties just as lists do. For example, the following properties indicate (using different metrics) whether or not the map is empty:
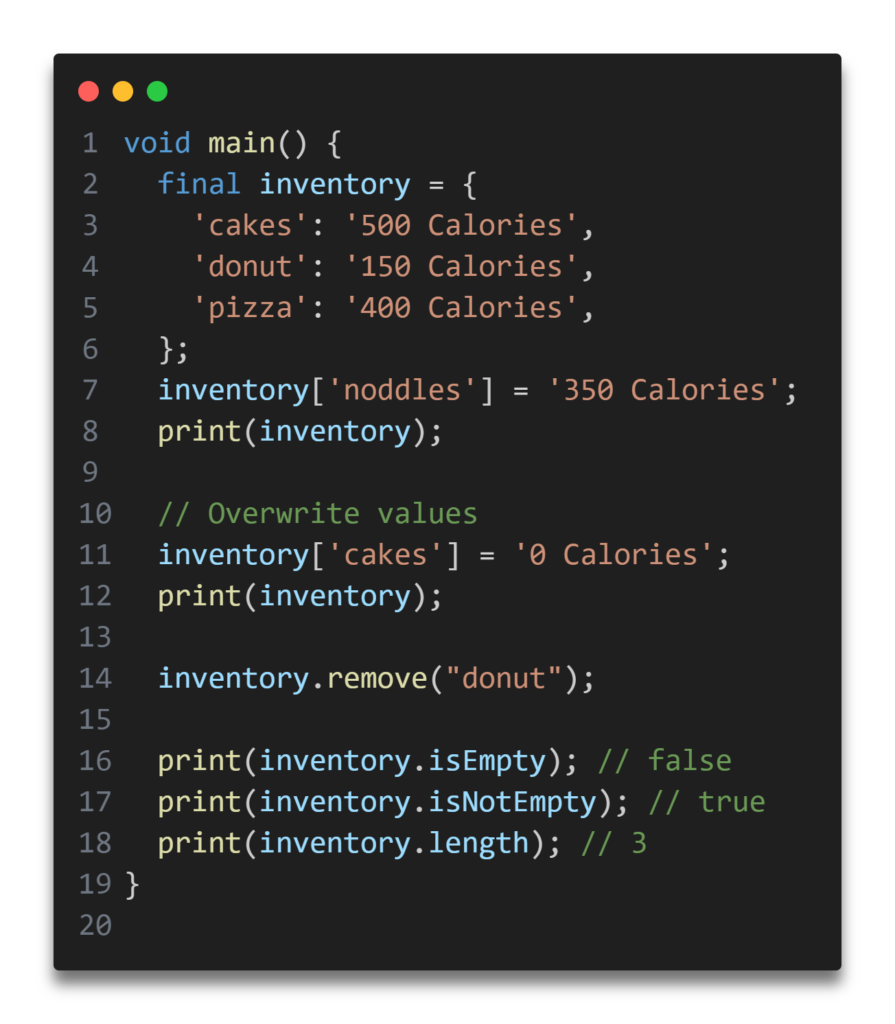
Checking for key or value existence
To check whether a key is in a map, you can use the containsKey method:
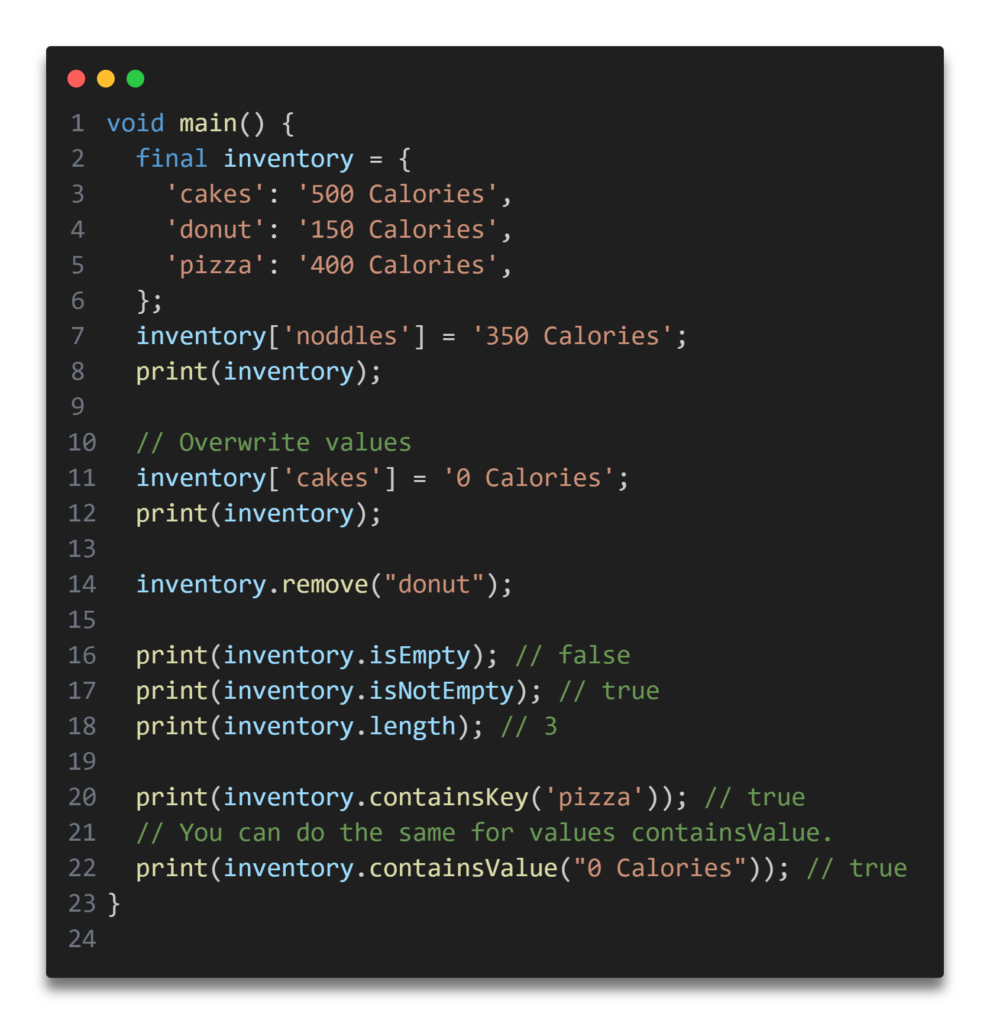
Thank you for taking the time to read! Feel free to explore more of our content or leave a comment below.
Check Algorithm Analysis too
Leave a Reply