Welcome, everyone!
In this article, we’ll dive into the Dart programming language. If you are new to Dart, this dart tutorial is for beginners.
Dart is a modern, object-oriented programming language developed by Google. It is designed for building web, server, and mobile applications.
With its clean syntax and powerful features, Dart is gaining popularity among developers, especially those working with Flutter for cross-platform mobile app development.
What’s Special About Dart?
The first question that come to my mind was,
What’s special about Dart?
Well, Dart is used in the flutter framework, which is entirely dependent on Dart.
So, for that reason, we need to learn Dart with great eagerness, and hunger like never before.
The first thing we will learn in Dart is its syntax.
If you have prior experience with any programming language or are familiar with C programming, you’ll know that some language, including C, have a main function as the starting point.
Similarly, Dart also has a main function we call the void main function, and the void main function look like this,
void main (){
print('Hello, Dart');
}
So, yeah did you notice something? I’m talking about its syntax.
Dart’s syntax is similar to C programming language, and its OOP concepts are inspired by Java, C#, and other programming languages.
Dart Core Concept
Let’s focus on the core concept of Dart.
The void main function —
It’s obvious that void means its return nothing.
The print statement is used to print the following text into the debug console.
Did you notice something when i said “print statement”?
The word statement is not just a word; it holds a lot of meaning. Keep this topic in mind, we will come back to it in some time.
Now you might be wondering how to create a project in Dart.
It’s not as simple as just opening VS code, creating a folder and writing code in a Dart file.
No, you have to follow the proper way to creating a new project.
In Dart, similar to flutter, just open a terminal and give the command.
dart create "yourProjectName"
Now hit the enter button.
You’ll see how its create a bunch of files and packages.
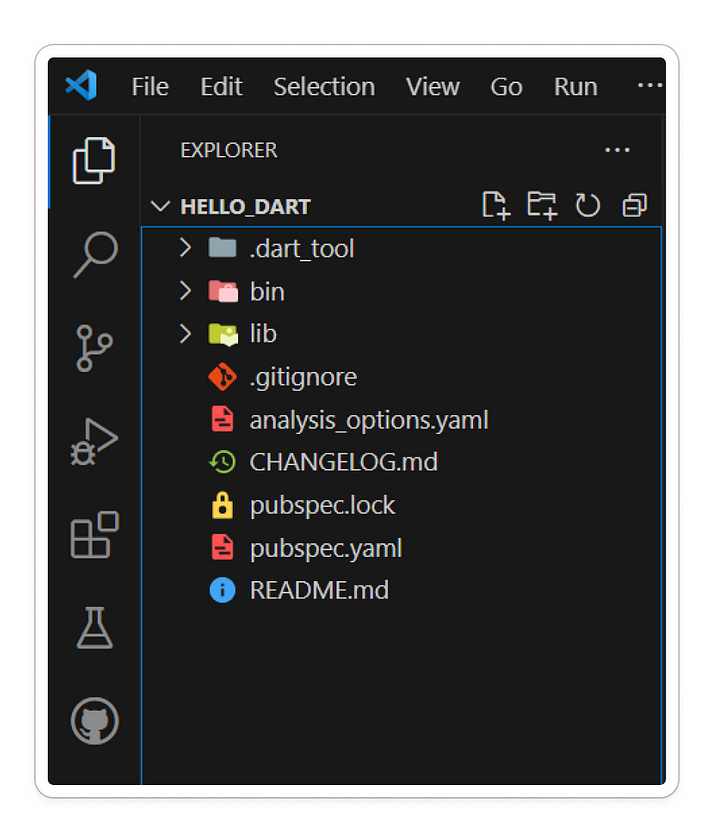
To run the project, use the following command.
dart run bin/yourProjectName.dart
You’ll see the text “Hello, World!” which is the output of the code in the default project that the create tool generated.
The “run” keyword is optional so you can run the project without it.
Again, “Hello, World!” is the result.
Structure and Contents of the Project
Take a look at the structure and contents of the Project.
The purpose of the main items in that folder are as follows:
- bin: Contains the executable Dart code.
- .gitignore: Formatted to exclude Dart — related files that you don’t need if you’re going to host your project on GitHub or another Git repository.
- analysis_options.yaml: Holds special rules that will help you detect issues with your code, a process know as linting.
- CHANGELOG.md: Holds a manually — curated Markdown — formatted list of the latest updates to your project.
Whenever you release a new version of a Dart project, you should let other developers know what you’ve changes. - README.md: Provide a basic (or not-so-basic) description of what your project does and how to use it.
Other developers will appreciate this greatly. - pubspec.yaml: Contains a list of the third-party Pub dependencies you want to use in your project.
The name pub spec stands for Pub specifications.
You also set the version number of your project in this file.
This is just a Simple Project
We are using a create tool above (dart create yourPorjectName) it created a simple console app because that’s the default.
However, you can create other types of projects using the
dart create --template console-full yourPorjectName
There are two additional directories lib and test.
In large projects, you’ll have many .dart files that you’ll organize under the lib folder.
You’ll also likely want to have tests to run against your Dart projects, and you can place those in the test folder.
Statement, Expression & Comment
Dart, like most other programming language, allows you to document your code through the use of comments.
These allow you to write any text directly along side your code and are ignored by the compiler.
The depth and complexity of the code you write can obscure the big-picture details of why you wrote your code a certain way, or even what problem it is your code is solving.
In simple terms.
Commenting your code in Dart is crucial for making it easier to understand, both for other and for your future self. Now next thing is.
How many types of comment in Dart?
So there are three types of comment in Dart.
- Single Comment
Use // for brief explanation like
int x = 10; //Initialize x with 10
- Multi-line Comment
Use /*…*/ for longer explanations. like
/*This is multi-line comment
It can span multiple lines.
*/
int y = 20;
- Documentation Comments
Use /// to describe classes, methods and variable in details.
These comment are used to generate document.
Documentation comments are super useful because you can
use them to generate…you guessed it…documentation!
You’ll want to add documentation comments to your code
whenever you have a public API so that the users, and your
future self, will know how your API works. Although this
book won’t go into depth on this, documentation comments
even support Markdown formatting so that you can add
elements like code examples or links to your comments.
This is all about Comment.
Well remember that i told you something about statement.
Now it’s time talk about statement.
Statement
Two important word that you’ll often here thrown about in programming langauge documentation are statement and expression.
It’s helpful to understand the difference between the difference the two.
Statement and Expression
So, Statement is a command something you tell the computer to do,
In Dart, all simple statements and with a semicolon.
You’ve already seen that with the print statement and this is use for simple statement.
print("Hello, World!");
Dart also has complex statements and code blocks that use curly braces, but there’s no need to add semicolons after the braces.
One example of a complex statement is the if statement. Again i repeat
No semicolons are needed on the lines with the opening or closing curly braces.
You’ll learn more about if statement in upcoming articles.
So now let’s talk about expression.
Expression
Unlike a statement an expression doesn’t do something it is something.
That is, an expression is a value, or is something that can be calculated as a value.
Here are a few example of expression in Dart.
42
3 + 2
'Hello World'
x
The values can be numbers, text, or some other type. The can even be variables such as x whose value isn’t know until runtime.
And the most important thing to manipulate this values we need to do some operations and each operation in Dart uses a symbol know as the operator to denote the type of operation it performs.
Consider the four arithmetic operations you learned in your early school days.
- Addition
- Subtraction
- Multiplication and
- Division
These operators are used like so.
2 + 6
10 - 2
2 * 4
24 / 3
Each of these lines is an expression because each can be calculated down to a value.
In these cases, all four expression have the same value : 8
Now listen carefully
All of the operations above use whole numbers, more formally know as integers.
However, as you know, not every number is whole, for example look at this.
22/7
If you want a integer division and expect the result to be 3.
However, Dart gives you the standard decimal answer.
3.14285714857143
If you actually did want to perform integer division then use truncated division.
22 ~/ 7
This produces a result of 3.
and one more thing you need to known.
The Euclidean modulo operation
Dart also has more complex operations you can use.
All of them are Standard mathematical operations, just not as common as the other.
You’ll take a look at them now.
The first of there is the Euclidean Modulo Operation.
That’s is complex name for an easy task.
In Division, The denominator goes into numerator a whole number of time, plus a remainder.
This remainder is what the Euclidean Modulo Operation calculates.
If you didn’t understand
Let’s break it down with an easy-to-understand example.
Imagine you have 10 Candies and you want to divide them equally among 3 friends, you give each friends 3 candies which uses up 9 of your 10 candies, you have 1 candy left over.
In mathematical terms:
- The denominator ( the number you’re dividing by ) is 3.
- The numerator ( the number you’re dividing) is 10.
- When you divide 10 by 3, you get 3 whole parts, and a remainder of 1.
The Euclidean Modulo operation is all about finding that remainder,
So, when you perform the Euclidean Modulo Operation with 10 and 3, you get 1, which is the left over candy in this example.
In Dart (the programming langauge), you can use the %
operator to find this remainder.
So, 10 % 3 would give you ‘1’,
showing that after dividing 10 by 3 you have a remainder of 1.
That’s all about modulo operator or you can say Euclidean modulo operation. Now next is Order of operation.
Order of operation
Of course, it’s likely that when you calculate a value, you’ll want to use multiple operation .
Here’s an example of how to do this in dart. ( (8000 / ( 5 * 10 ) ) — 32 ) ~/ (29 % 5 )
When you perform mathematical calculations, the order in which you do the operation matters.
This is often remembered by the acronym PEMDAS (Parentheses, Exponents, Multiplication and Division, Addition and Subtraction ) from left to right.
Here’s how Dart (or any standard math rule) processes this.
- Parentheses first
Always do the operations inside parentheses first.
– Innermost Parentheses
( 5 * 10 ) equal to 50.
– Substitute back into the expression
( 8000 / 50 ) - Division Next
Now handle the division inside the remaining parentheses.
( 8000 / 50 ) equals to 160.
– Substitute back into the expression ( 160 — 32 ) - Now perform the subtraction inside the parentheses.
160–32 equal 128
– Substitute back into the expression 128 ~/ ( 29 % 5 ) - Now perform the modulo operation next.
29 % 5 means you divide 23 by 5 and find the remainder.
Since 29 divide by 5 is 5 with remainder 4,
29 % 5 equals 4.
– Substitute back into the expression
128 ~/ 4 equal to 32.
Finally, perform the integer division denoted by ~/ in Dart.
So That’s all for today! Don’t forget to Like and Follow to stay updated. Happy Coding!.
Hi, this is a comment.
To get started with moderating, editing, and deleting comments, please visit the Comments screen in the dashboard.
Commenter avatars come from Gravatar.